List of Javadoc Block Tags
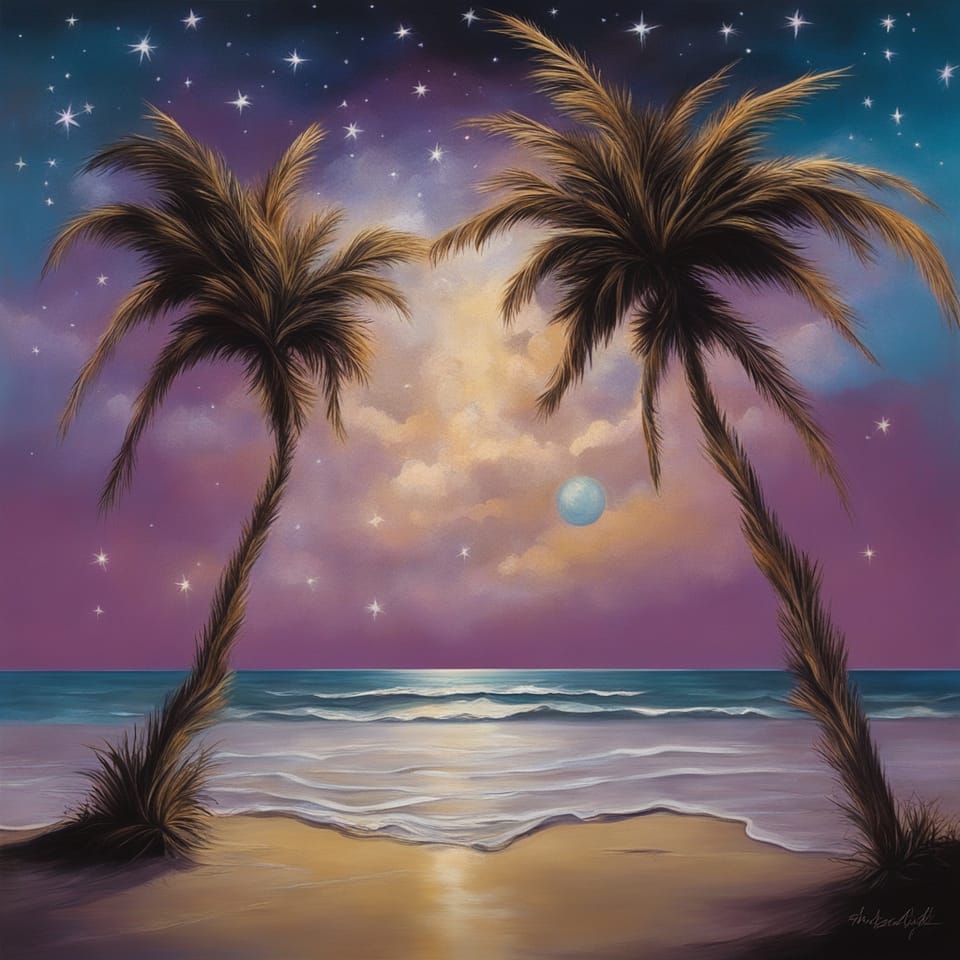
Block Tags
Block tags are tags used in the tag section of a Javadoc. Javadocs start with a main description. After the description is the tag section.
Common Block Tags
Common block tags are block tags that will always apply when writing a Javadoc comment.
@param
Usages: constructors and methods.
The @param tag is used to document parameters. There is a @param tag for each parameter. A @param tag starts with the parameter name, followed by the description of the parameter.
/**
* (description...)
*
* @param firstName The name placed at the beginning
* @param lastName The name placed at the end
*/
public void method(
final String firstName,
final String lastName) {
// ...
}
@return
Usages: methods.
A @return tag is used to describe what is returned from a method. This is only used on methods that do not return void.
/**
* (description...)
*
* @return A number between 1-10
*/
public int random() {
// ...
}
@throws
Usages: constructors and methods.
The @throws tag is used to document the exceptions defined in a throws clause. This starts with the exception class that is thrown, followed by a description of when it is thrown. There is a @throws tag for each exception defined in a throws clause.
/**
* (description...)
*
* @throws MyException when user is not logged in
* @throws IOException when file is not found
*/
public void method() throws MyException, IOException {
// ...
}
When generating a Javadoc, if there isn't a @throws tag and there is a throws clause on a method, it will still show the exception(s) in the throws clause in the generated output. If you aren't going to provide a description, you might as well omit this tag.
@see
Usages: anywhere.
The @see tag is used to link to other content. You can have zero or more @see tags. A @see tag can come in three different forms.
The first is just a description. This is written like a String value.
/**
* (description...)
*
* @see "Byte-size"
*/
public void random() {
// ...
}
The second is a URL (Uniform Resource Locator).
/**
* (description...)
*
* @see <a href="www.bytesize.press">Byte-size</a>
*/
public void random() {
// ...
}
The third is referencing a package, class, interface, method, or field. This has an optional label at the end. If the label is provided, it will show the label. If there isn't a label provided, it will just show the name.
/**
* (description...)
*
* @see MyClass LabelToDisplay
* @see MyClass#method
*/
public void random() {
// ...
}
This will show the following output:
LabelToDisplay
MyClass#method
@since
Usages: anywhere.
The @since tag is used to specify the version it was added.
/**
* (description...)
*
* @since 6.1
*/
public void random() {
// ...
}
This is an optional tag.
@deprecated
Usages: anywhere.
The @deprecated tag is used to mark something as deprecated and provide a description of when it was deprecated and what to use instead. The format for the description should look like the following:
/**
* (description...)
*
* @deprecated As of version 5, replaced by
* {@link #newMethod(String, String)}
*/
public void oldMethod(
final String param1,
final String param2) {
// ...
}
Uncommon Block Tags
Uncommon block tags are block tags that are still in use, but the usage is fading because there is a better way of doing things in modern times. Depending on the tag, this may be better done using an annotation similar to using the @Override annotation or using your SCCS (Source Code Control System) instead.
@author
Usages: packages, classes, and interfaces.
The @author tag is used to document who the author is. This tag can be used for a single author or multiple authors comma separated.
/**
* (description...)
*
* @author John Doe, Jane Doe
*/
public class MyClass {
}
You can also use more than one @author tag instead of comma-separated multiple authors.
/**
* (description...)
*
* @author John Doe
* @author Jane Doe
*/
public class MyClass {
}
When generating a Javadoc, this will not be included using the default options. This tag is generally only used in your SCCS. Your SCCS is better to use than this tag.
@version
Usages: packages, interfaces, and classes.
The @version tag is used for the current version.
/**
* (description...)
*
* @version 1.5
*/
public class MyClass {
}
A @version tag can be populated when the code is checked out of your SCCS. Each SCCS is different in how you would write this. The version tag is used less and less since the source control system handles this better.
Don't get the @version tag confused with the @since tag. The @since tag is used for what version it was introduced.
@exception
This is an old tag and is identical to the @throws tag. The @throws tag should be used instead.
Order of Block Tags
When using block tags, the standard is to order the tags as the following:
@author
@version
@param
@return
@throws/@exception
@see
@since
@serial
@deprecated
Conclusion
Block tags are used in the tags section of a Javadoc. What can be used and what should be used depends on what you are documenting. Javadoc also contains the tags @serial, @serialData, and @serialField for serializing data. They aren't commonly used, so they aren't covered here.