List of Javadoc Inline Tags
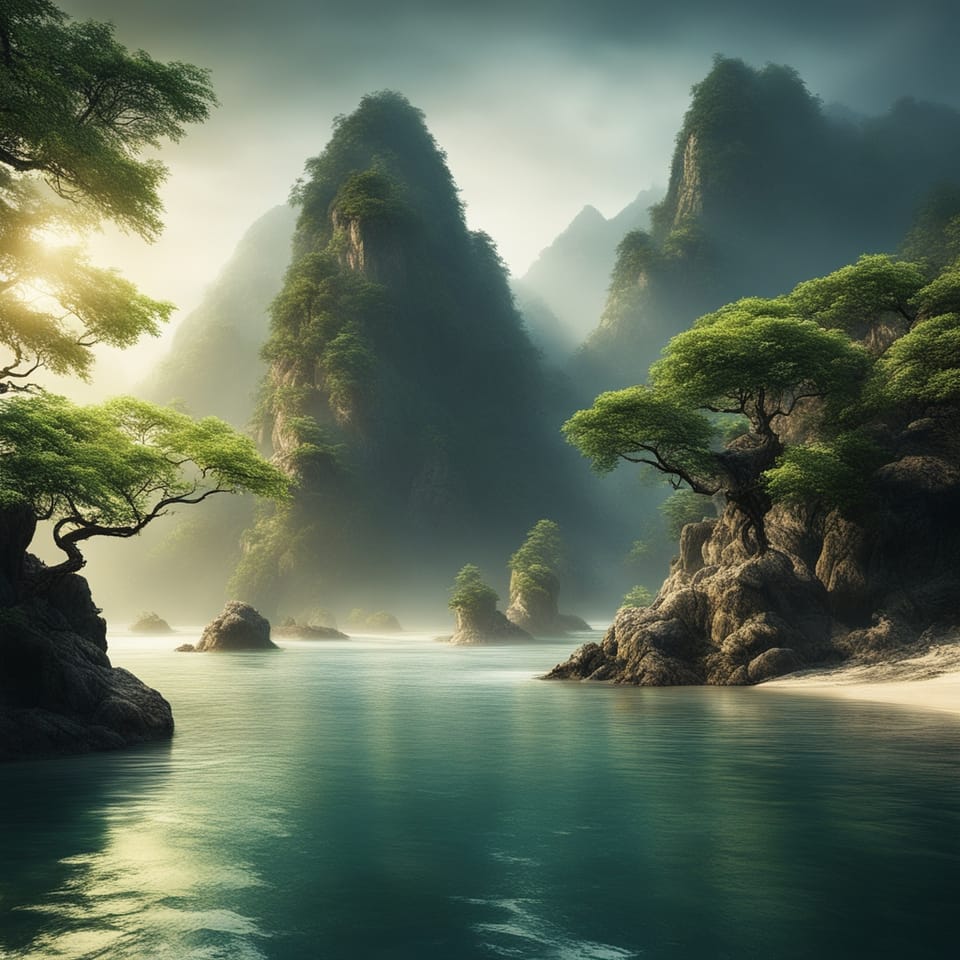
Inline Tags
Inline tags are tags used in descriptions. This can be the main description in a Javadoc or a tag's description. Inline tags follow the same syntax as block tags but are enclosed in {}. Inline tags can be used in any Javadoc comment or block tag with the exception of @{inheritDoc}.
{@code}
The @code tag is used to display text using code text font.
/**
* Method to replace {@code !isEmpty(value)}
*
* @param value ...
* @return ...
*/
public boolean isNotEmpty(final String value) {
// ...
}
This is the same as putting code in HTML with the following:
/**
* Method to replace <code>!isEmpty(value)</code>
*
* @param value ...
* @return ...
*/
public boolean isNotEmpty(final String value) {
// ...
}
{@docRoot}
The @docRoot tag is used to represent the relative path to the generated Javadoc. It is used to include pages such as a copyright page, a license page, or images such as a logo.
package press.bytesize;
/**
* See the <a href="{@docRoot}/license.html">License</a>.
*/
public class MyClass {
}
Since this class is the package press.bytesize, the path to MyClass would be /press/bytesize/MyClass.java. The path in the previous example will resolve to the following:
<a href="../../license.html">
{@inheritDoc}
The @inheritDoc tag is used to copy a Javadoc. This will use the nearest implementable interface or inherited classes Javadoc.
public interface Nullable {
/**
* Test an object to see if it is null or not.
*
* @param object The object to check if it is null
* @return True if the object is null and false if it is not null
*/
boolean isNull(final Object object);
}
public class MyClass implements Nullable {
/**
* {@inheritDoc}
*/
@Override
public boolean isNull(Object object) {
// ...
}
}
The Javadoc for isNull(Object) in MyClass will use the same Javadoc that is on isNull(Object) in Nullable.
You can also use it to copy the description from the block tags @param, @return, and @throws.
public class MyClass implements Nullable {
/**
* New description instead of the description in Nullable
*
* @param object {@inheritDoc}
* @return {@inheritDoc}
*/
@Override
public boolean isNull(Object object) {
// ...
}
}
This will have a new main description, and copy the description for the @param tag and the @return tag.
{@link}
The link tag is used to link to another class, method, field, or URL the same way you use a @see block tag. The difference between the @link tag and the @see block tag is that @link can be inline.
/**
* Also checkout {@link MyClass#method}
*/
{@linkplain}
The @linkplain tag is the same as the @link tag except it displays the text in plain text font instead of using code text font.
{@literal}
The literal tag is used to display the literal value inside the tag. It is used so Javadoc tags and HTML are not interpreted.
/**
* Text with HTML {@literal <code></code>}
* and Javadoc tags {@literal @return}
*/
This will produce the following output:
Text with HTML <code></code> and Javadoc tags @return
{@value}
The @value tag is used to display a constant's value. Using it on a constant can be done with the following:
public class Table {
/**
* This value is @{value}
*/
public static final int HEADER_INDEX = 0;
}
This will produce the following text:
This value is 0
This can also be used on any Javadoc comment to display a constant's value.
public class MyClass {
/**
* Header index value is {@value Table#HEADER_INDEX}
*/
public void method() {
}
}
Conclusion
Inline tags can be used anywhere in Javadoc comments or block tags with the exception of @{inheritDoc}. They are used in main descriptions and block tag descriptions.