ListIterator in Java
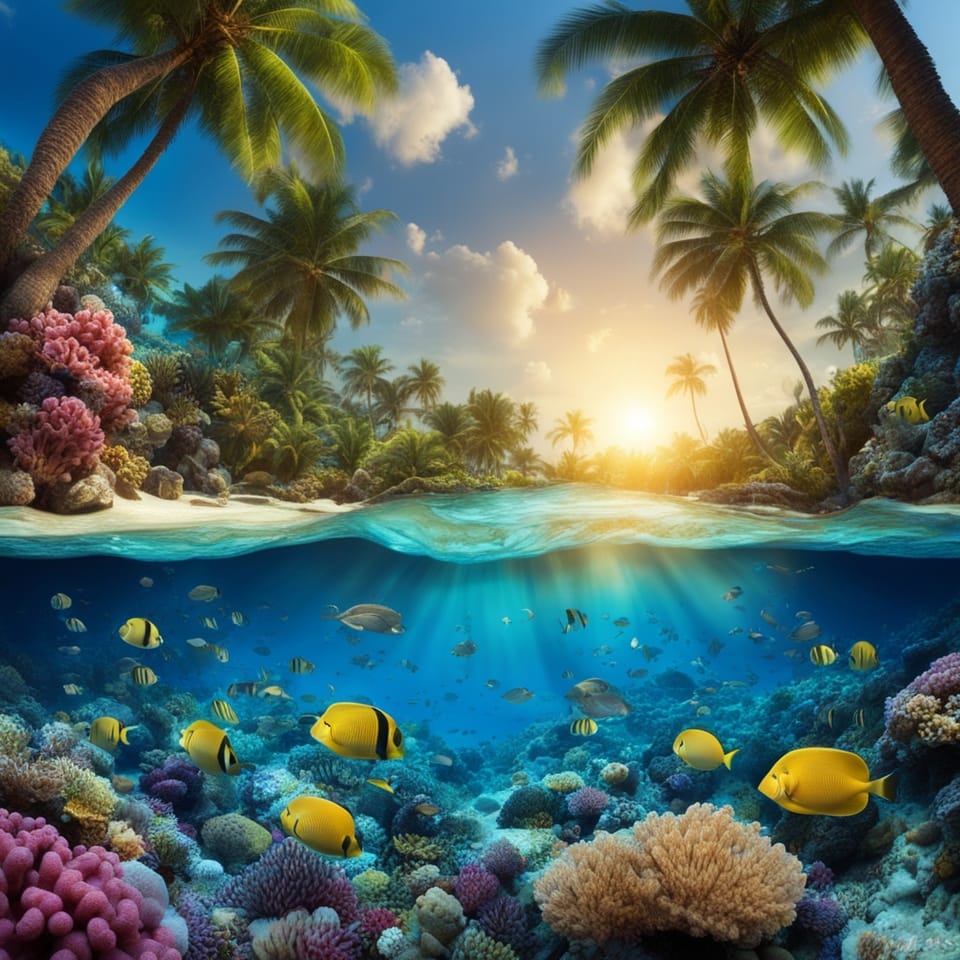
What is a ListIterator?
A ListIterator is a bidirectional iterator that allows you to move the iterator cursor position forward or backward. The List interface has two factory methods for providing a ListIterator implementation. The difference between these two methods is the starting cursor position. One starts at the beginning cursor position, and the other allows you to specify a starting cursor position. These methods on the List interface look like the following:
public interface List<E> {
// ...
ListIterator<E> listIterator();
ListIterator<E> listIterator(int index);
// ...
}
The listIterator(int) method would return the element at the specified index when the next() method is called.
ListIterator Methods
The ListIterator interface extends the Iterator interface. It has the following methods:
public interface ListIterator<E> {
// Query operations
E next();
E previous();
boolean hasNext();
boolean hasPrevious();
int nextIndex();
int previousIndex();
// Modification operations
void add(E e);
void remove();
void set(E e);
}
Next and Previous Elements
ListIterator doesn't have a cursor position. Instead, the cursor position is determined by what next() and previous() will return.
You can get the next element using the next() method.
final var next = listIterator.next();
You can get the previous element using the previous method.
final var previous listIterator.previous();
If the previous() method is called when the cursor position is at the starting position, it will throw a NoSuchElementException.
Has Methods
The has methods will return true if there is a previous or next element and false if they do not.
If you are wanting to move the cursor position forward, you can check to see if there is a next element with the hasNext() method.
listIterator.hasNext();
If you are wanting to move the cursor position backwards, you can check to see if there is a previous element with the hasPrevious() method.
listIterator.hasPrevious();
If hasPrevious() is called on the first element, it will return false.
Index Methods
When using a ListIterator, you have the ability to get the previous and next index. You can get the next element index using the nextIndex() method.
final int nextIndex = listIterator.nextIndex();
If the cursor position is at the last position, nextIndex() will return the last index.
You can get the previous element index using the previousIndex() method.
final int previousIndex = listIterator.previousIndex();
If previousIndex() is called on the first element, it will return -1.
Modifications Methods
There are multiple modification methods that can be used to add, remove, or replace the elements in a ListIterator. To add an element to the next iteration of the ListIterator, you use the add() method.
listIterator.add(8);
You can remove the current element obtained with either next() or previous() using the remove() method.
listIterator.remove();
You can replace the current element with a new value using the set() method. This will be the element that pertains to the last call to previous() or next().
listIterator.set(8);
Conclusion
ListIterators are implemented on the List interface. They are a bidirectional iterator that allows you to add, replace, or remove at the cursor position you are in.