Lists in Java
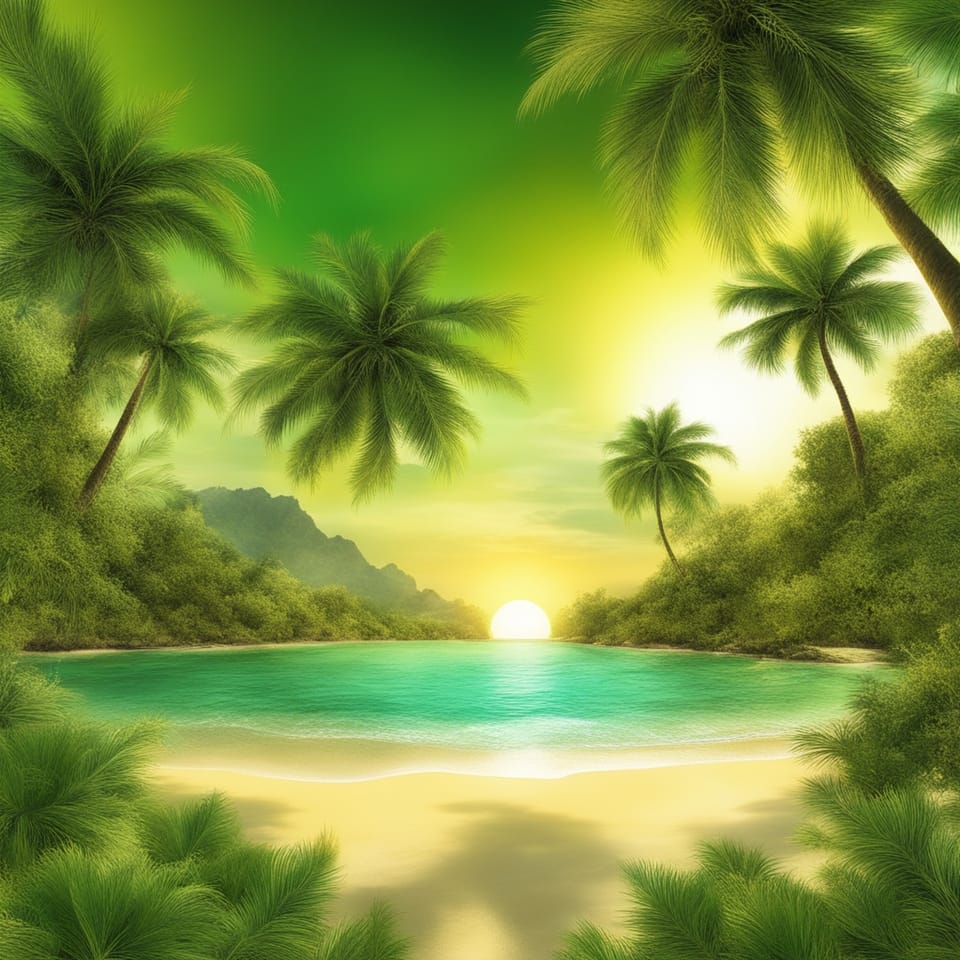
Lists
Lists are ordered, non-unique collections that can contain null elements. Lists can grow in size and do not have a fixed size like an array does.
Lists in Java will usually use the ArrayList implementation. Although Java contains several List implementations, the ArrayList is a highly optimized implementation and can usually outperform other implementations. An ArrayList implements the following interfaces:
- java.util.List
- java.util.SequencedCollection
- java.util.Collection
- java.lang.Iterable
- java.util.RandomAccess
- java.lang.Cloneable
- java.io.Serializable
Any methods that take an index parameter will throw an IndexOutOfBoundsException if that index doesn't exist.
ArrayList Constructors
The no argument constructor initializes the ArrayList with a capacity of 10.
final List<Integer> numbers = new ArrayList<>();
If you want to specify the initial capacity, you can provide that value with the following:
final List<Integer> numbers = new ArrayList<>(100);
You can also create an ArrayList and add the elements from another collection to it.
final List<Integer> numbers = new ArrayList<>(collection);
Static Factory Methods
The List.of() method builds a list of non-null elements. This method doesn't return an ArrayList but instead returns some type of unmodifiable list implementation depending on which overloaded method is called.
// Since Java 9
final List<Integer> unmodifiableList = List.of(1, 2, 3);
The List.copyOf() method takes a collection and converts it to an unmodifiable list. The collection cannot be null, and the collection cannot have null elements.
// Since Java 10
final List<Integer> unmodifiableList = List.copyOf(collection);
Modification Methods
The add() method adds an element to the end of the list. This returns true if the element was added and false if it wasn't.
list.add("blue");
You can add an element at a specific index using the following:
list.add(2, "blue");
This adds an element at index 2.
The addFirst() method adds an element at the beginning of the list.
list.addFirst("blue");
The addLast() method adds an element at the end of the list.
list.addLast("blue");
The remove() method removes an element. This returns true if the element was removed and false if it wasn't.
list.remove("blue");
You can remove an element at a specific index using the following method:
list.remove(2);
This will remove the element at index 2.
Positional Access Methods
The get() method allows you to get an element at a specific index.
final String color = list.get(2);
The getFirst() method gets the first element in the list.
final var firstElement = list.getFirst();
The getLast() method gets the last element in the list.
final var lastElement = list.getLast();
The set() method allows you to replace an element at a specific index.
final String previousValue = list.set(2, "blue");
This replaces the element at index 2 and returns the element that was previously at that index.
Iterating
Since lists implement the Iterable interface, you can iterate over the elements in a list using a foreach loop.
for (final Integer element : numbers) {
// ...
}
There is also a forEach() method to iterate over the elements.
numbers.forEach(
number -> // ...
)
Query Methods
The size() method returns the total number of elements in the list.
final int size = list.size();
The isEmpty() method checks to see if there are any elements in the list. This returns true if there are zero elements and false if there are one or more.
if (list.isEmpty()) {
// ...
}
The contains() method takes an object and returns true if the element is in the list and false if it is not. This uses the equals() method to check for equality.
public boolean contains(E e);
Bulk Operations Methods
Bulk operations work with a Collection of elements. When the size of a list is increasing, using a bulk operation will increase the performance of adding those elements instead of adding them one by one. The methods that return a boolean will return true if the size of the list changed and false if it didn't. Any of the methods that have all in the name will throw a NullPointerException if a null collection is passed to it.
The addAll() method will add a collection of elements at the end of the list.
list.addAll(collection);
You can insert a collection at a specific index with the following:
list.addAll(2, collection);
This inserts all the elements in the collection variable starting at index 2.
The removeAll() method removes all elements in a collection.
list.removeAll(collection);
The retainAll() method removes all elements that aren't in the collection provided.
list.retainAll(collection);
The containsAll() method checks to see if all the elements in a collection are contained in the list. This will return true if all elements are contained in the list and false if not all of them are contained in the list.
final boolean allElementsAreInList = list.containsAll(collection);
The clear() method removes all elements from a list.
list.clear();
The sort() method changes the order of the elements in a list using a Comparator to sort the list.
numbers.sort(Integer::compareTo);
Search Methods
The indexOf() method finds the first occurrence of an element.
final int firstIndex = list.indexOf("blue");
The lastIndexOf() method finds the last occurrence of an element.
final int lastIndex = list.lastIndexOf("blue");
Factory Methods
The toArray() method converts a list into an Object array.
final Object[] array = list.toArray();
Usually when you are converting to an array, you are going to want to maintain the type. You can do this by specifying the type by passing in an empty array of the type.
final Integer[] array = numbers.toArray(new Integer[0]);
Java 11 introduced a way to convert to an array that makes more sense and should be used instead.
final Integer[] array = numbers.toArray(Integer[]::new);
The subList() method creates a new list of a subset of elements. This takes a starting index (inclusive) and an ending index (exclusive).
final List<Integer> numbers = List.of(1, 2, 3, 4, 5, 6, 7, 8, 9);
final List<Integer> subList = numbers.subList(1, 5);
The subList variable will contain the elements [2, 3, 4, 5].
The clone() method makes a new copy of a list.
final List<String> newList = list.clone();
The iterator() method converts a list into an iterator().
final Iterator<String> iterator = list.iterator();
The listIterator() method converts a list into a ListIterator.
final ListIterator<String> listIterator = list.listIterator();
You can also specify a starting index and create a ListIterator that starts at that index.
final ListIterator<String> subListIterator = list.listIterator(3);
The spliterator() method converts a list into a Spliterator.
final Spliterator<String> spliterator = list.spliterator();
The stream() method converts a list into a Stream.
final Stream<String> stream = list.stream();
The parallelStream() method converts a list into a Stream that can be processed in parallel.
final Stream<String> stream = list.parallelStream();
Memory Methods
There are methods to manage the memory on a list. These methods are not commonly used because ArrayList already does this well, but there are still use cases where you'd need them.
The ensureCapacity() method will increase the capacity of a list.
list.ensureCapacity(1000);
The trimToSize() method shrinks the capacity to how many elements are in the list.
list.trimToSize()
Object Methods
The equals() method will use the Objects.equals() method on each element to determine equality.
final boolean isEquals = list1.equals(list2);
The hashCode() method returns the hashCode of the list.
final int hashCode = list.hashCode();
The toString() method will call toString() on each element in the list and build a String of those values.
System.out.println(
List.of(1, 2, 3);
);
This will print [1, 2, 3] to the console.
Conclusion
Lists are used to provide an ordered collection where elements can be null and non-unique. Lists are probably the most commonly used collection.