Local Classes in Java
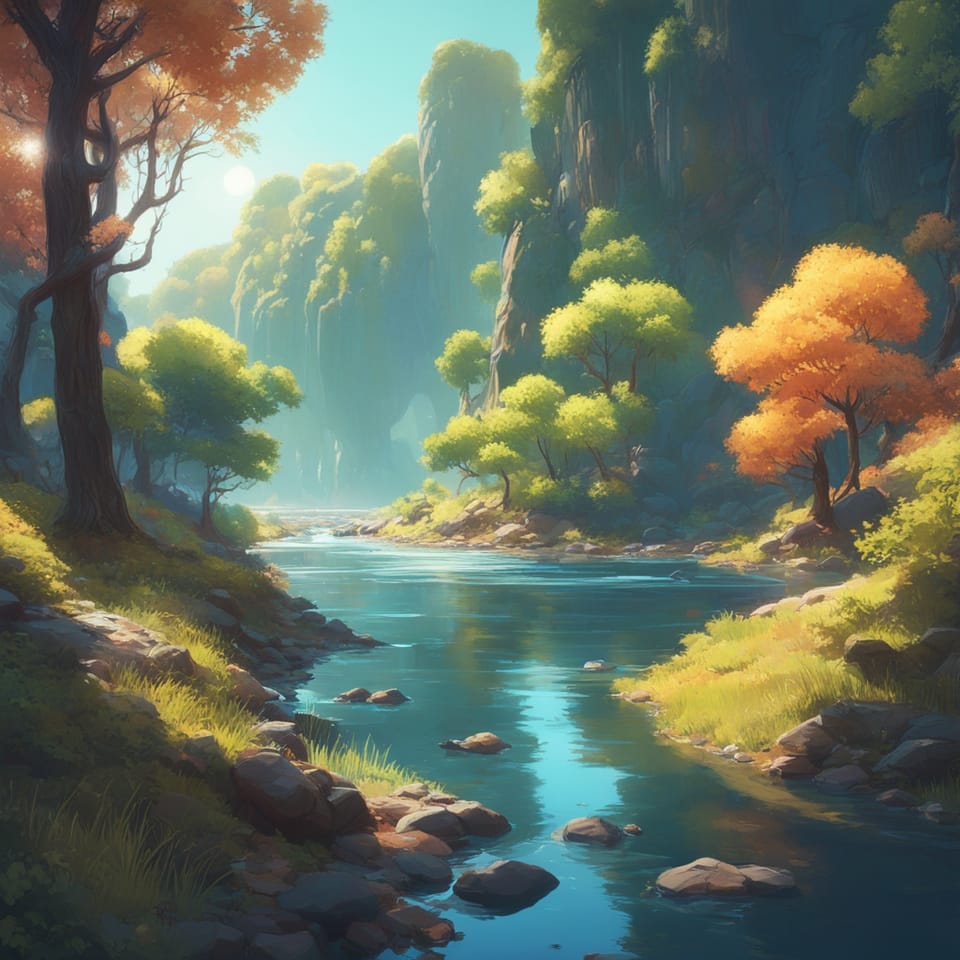
Local Classes
Local classes are classes defined inside of a method. They are written just like a normal class and are a way to encapsulate a class that is only used in a method. Local classes can access variables in the method they are declared in. They can also access methods and variables in the class the method is in, just like an inner class. An example of a local class is the following:
public class MyClass {
public void method() {
class LocalClass {
// ...
}
}
}
Encapsulation and Local Classes
Local classes offer the ultimate form of encapsulation. Not only are local classes encapsulated in the method they are declared in, but the scope of where an instance can be created can be restricted to a block such as an if statement, loops, etc. The scope of where the local class can be created follows the same rules as a variable in a method.
public void method() {
if (condition) {
class MyClass {
// ...
}
// ...
}
}
In this example, the local class is defined inside the if block. The scope of where instances of MyClass can be created is only in the if block. If you try to create an instance of MyClass outside of the if block, you will get a compiler error.
Anonymous Classes
Anonymous classes are unnamed classes. You can only create one instance of it. One way to get around this is to create a method that returns an instance of an anonymous class.
public MyClass create() {
// Anonymous class
return new MyClass() {
// ...
}
}
With the use of a local class, they are named, so you can create multiple instances of that local class inside the method it is declared in.
Polymorphism
Local classes cannot be used as a return type in a method since they are local to the method. If you try to do this, you will get a compiler error.
// Compiler error:
// cannot find symbol class MyClass
public MyClass create() {
class MyClass {
}
return new MyClass();
}
When using polymorphism, you can return the interface the local class implements or the class it extends.
public interface Parser {
String parse(final byte[] bytes);
}
public Parser mockParser() {
class MockParser implements Parser {
@Override
public String parse(final byte[] bytes) {
return "";
}
}
return new MockParser();
}
In this example, the method returns an instance of Parser. Inside the mockParser() method, a local class is declared, and an instance of it is returned from the method.
Conclusion
Local classes are a way to encapsulate a class inside of a method. They can be defined anywhere in a method and follow the same scope as a variable and where it can be used. Although they are something that isn't going to be common to use, being aware of them when you need them is valuable.