Marker Interfaces in Java
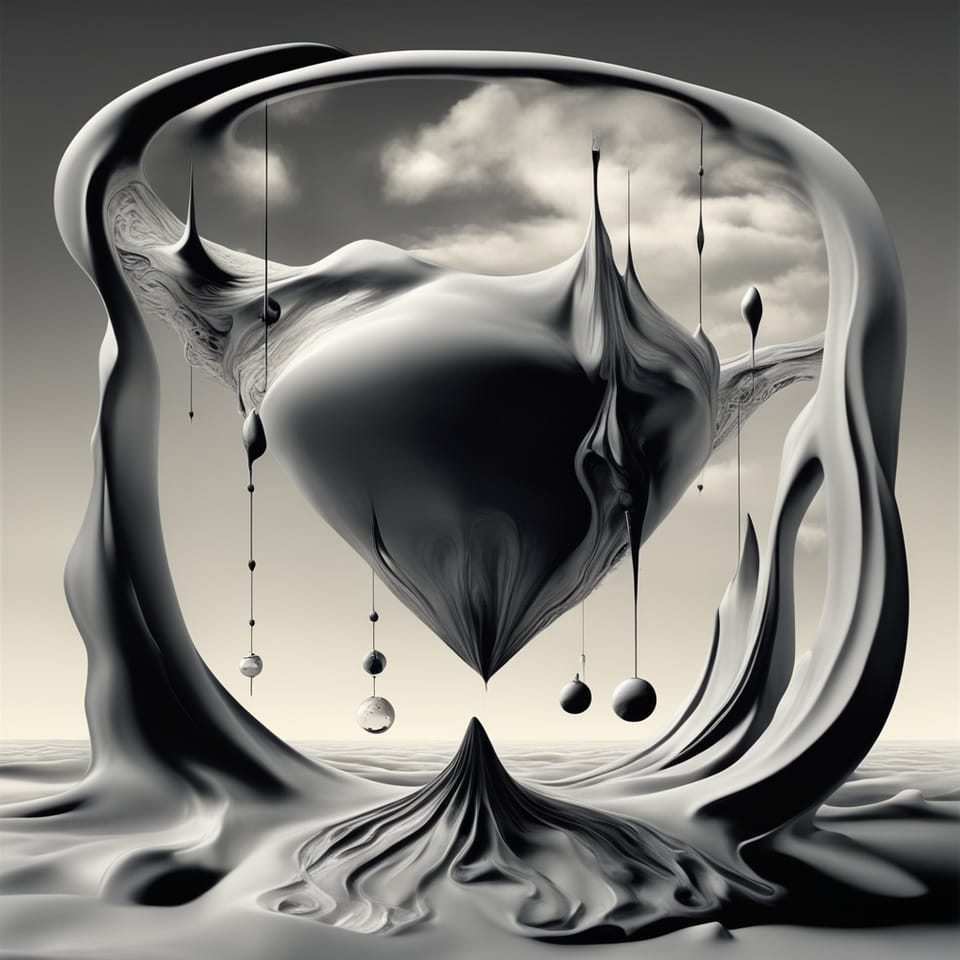
Marker Interfaces
Marker interfaces are used to provide metadata to a class or interface. A marker interface is an empty interface that doesn't contain any methods or constants.
public interface MyMarkerInterface {
}
An example of a marker interface that is built into Java is the java.io.Serializable interface. This is used to serialize and deserialize classes that implement this interface. If you try to serialize a class and it doesn't implement the java.io.Serializable interface, a java.io.NotSerializableException will be thrown.
Marker interfaces introduce a new type, so it allows you to use something more restrictive than the Object class. For example:
// Marker interface
public interface Persistable {
}
public class GenericDao {
public Optional<Persistable> findById(final Serializable id) {
// ...
}
public void insert(final Persistable persistable) {
// ...
}
public void update(final Persistable persistable) {
// ...
}
public void delete(final Persistable persistable) {
// ...
}
}
If these methods were using Object instead of the Persistable marker interface, anything could be passed to it, which doesn't make sense.
Marker Annotations
Annotations were introduced in Java 5. Using annotations in a lot of cases is a better option than using a marker interface. There are some key differences between the two. Annotations aren't types but a runtime feature using reflection. Because of this, they can push potential errors off until runtime. Marker interfaces introduce a new type. This allows the compiler to catch potential errors at compile time.
Conclusion
Marker interfaces introduce a way to tag an interface or class with a type. If you do not need a type, using an annotation is probably the better approach.