Method Overriding vs Method Overloading
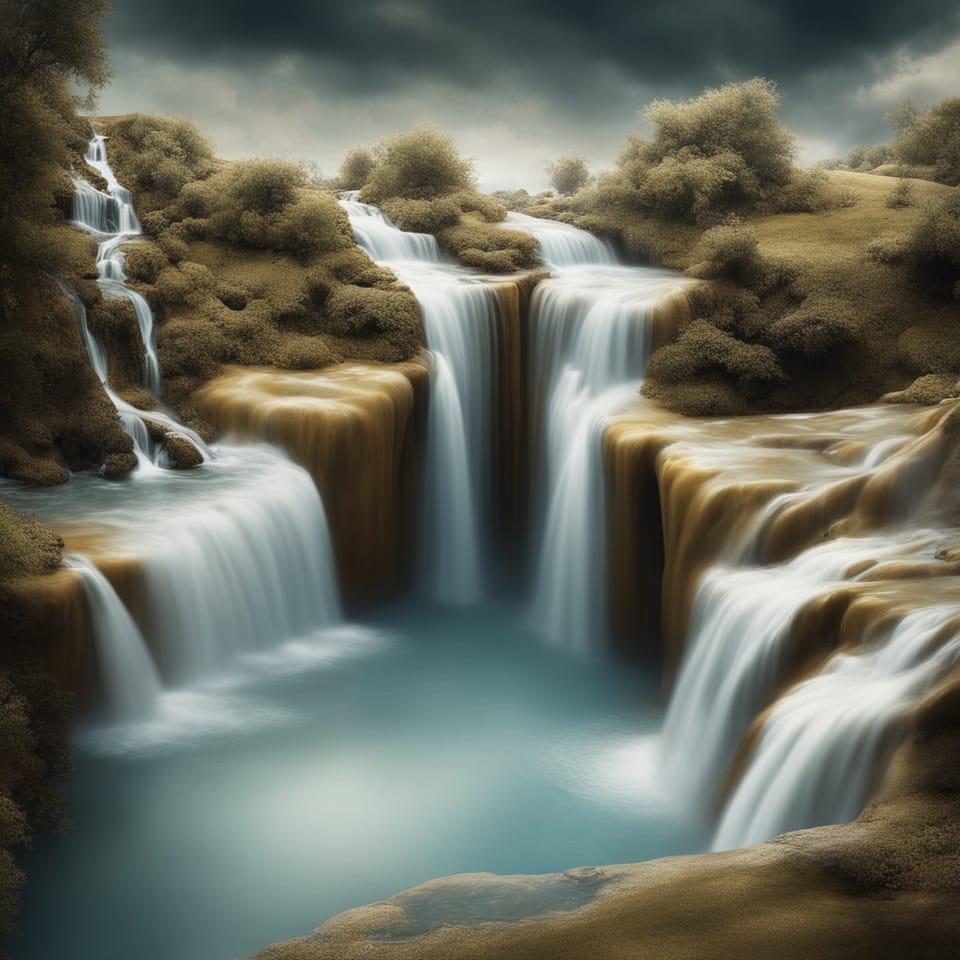
Method Overriding
Method overriding is used when you are working with inheritance. Method overriding is where you change the implementation of a method in a subclass that has an implementation provided in a superclass. An overridden method will have the same return type, the same parameters, and the same method name. For example, take the following class.
// Java code
public class Animal {
public void jump() {
System.out.println("Jump...");
}
}
An example of a subclass that inherits the Animal class may look like the following:
// Java code
public class Fish extends Animal {
// ...
}
It doesn't make sense that a fish could jump. So in this case, the jump method would be overridden to provide a different implementation of it.
// Java code
public class Fish extends Animal {
@Override
public void jump() {
throws new RuntimeException("Unsupported method");
}
}
This is an example of method overriding.
Method Overloading
Method overriding is where you have multiple methods with the same name but with different parameters. The method parameters can be different data types and/or have a different number of parameters. An example of method overloading would be the following:
public class Example {
public void print(final int number) {
System.out.println(number);
}
public void print(final double number) {
System.out.println(number);
}
public void print(
final String firstName,
final String lastName) {
System.out.println(firstName + " " + lastName);
}
}
This has three methods, all named print. Each of these also returns void. The difference is the parameters that each method takes.
Conclusion
Method overriding is where you provide a different implementation of a method in a subclass where there is already an implementation in the superclass. Method overloading is where you have the same method name but have different method parameters.