Multi-line Strings in Swift
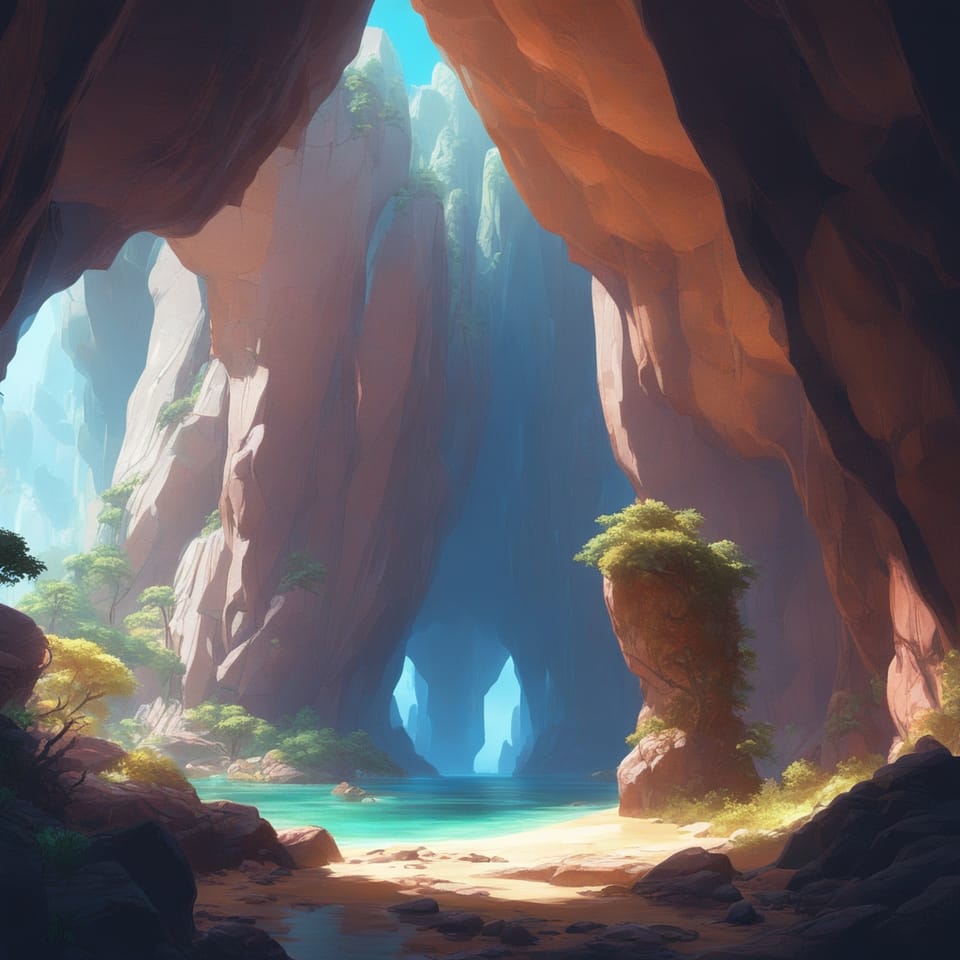
Multi-line Strings
When creating a string, they are usually just single-line strings. If you want to create a block of text in your string, it can be difficult with string concatenation. This is where multi-line strings come into play.
Multi-line strings are defined using a triple double quote and an ending triple double quote.
let html = """
<html>
<head>
</head>
<body>
</body>
</html>
"""
This will generate a String with the following value. Spaces have been replaced with periods.
<html>
....<head>
....</head>
....<body>
....</body>
</html>
The previous multi-line string is equivalent to the following using string concatenation:
let html =
"<html>\n" +
" <head>\n" +
" </head>\n" +
" <body>\n" +
" </body>\n" +
"</html>" +
Where the indentation of the value in the text block ends determines where the indentation starts.
let value = """
Hello
World
""";
This will result in the example below. The spaces have been replaced with periods.
Hello
....World
Moving the ending three double-quotes like the following:
let value = """
Hello
World
"""
This will result in the example below. The spaces have been replaced by periods.
........Hello
....World
If the indentation of the last triple double quotes is more than the value of the String, it will result in a compiler error.
// Compiler error:
// note: change indentation of this line to match closing delimier
let value = """
Hello
World
"""
Preventing Line Breaks
If you want to build a single-line string using a multi-line String, this can be done by providing a \ at the end of a multi-line string. This comes at the end of the line of a multi-line String.
let value = """
A multi-line \
string as \
a single line
"""
This will produce the following value:
A multi-line string as a single line
Conclusion
Multi-line strings are great when you have a large string value or a string with line breaks that you need to format. They can be used both when you need line breaks or when you don't need line breaks.