Mutable vs Immutable
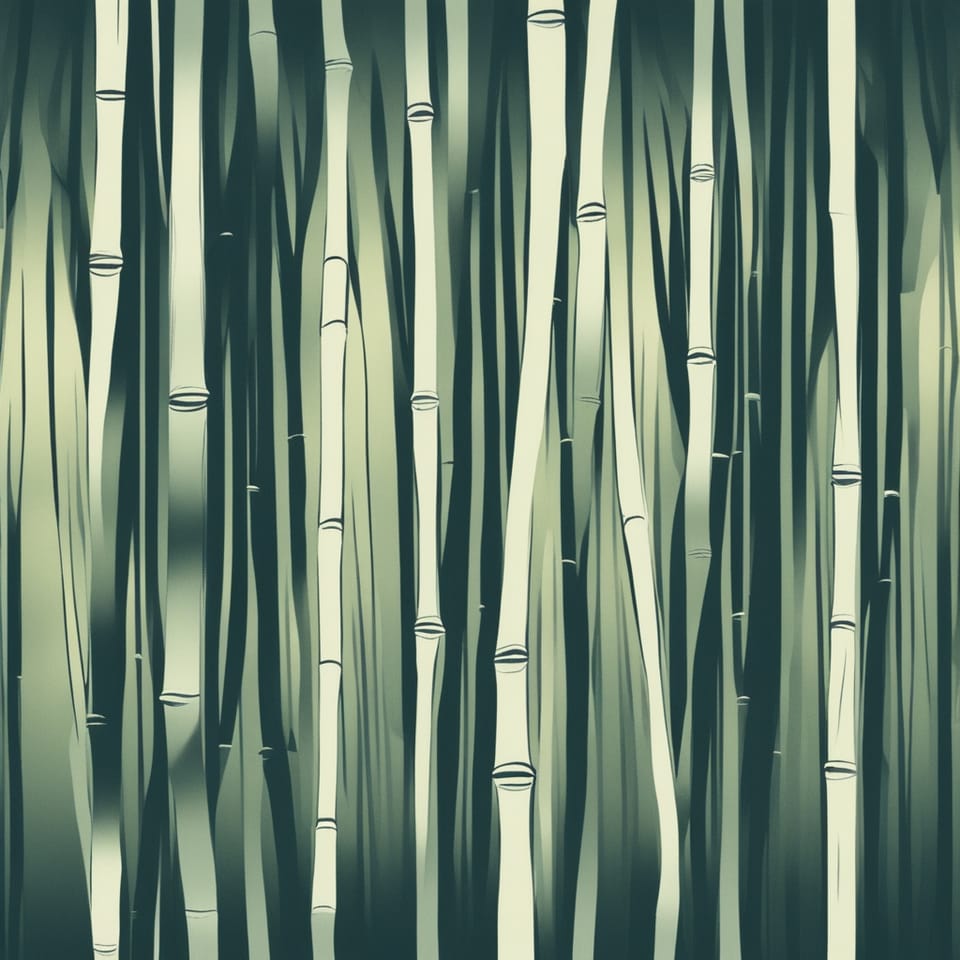
Mutable Variables
Mutable variables are variables that can be reassigned a value after they have been assigned a value.
// Java code
// Mutable index
int index = 0;
index = 1;
index = 2;
A mutable variable is used for storing modifiable state, such as a counter in a loop.
Immutable Variables
Immutable variables are variables that cannot be reassigned after they have been assigned a value. In the Java programming language, immutable variables are declared with the final keyword.
// Java code
// Immutable index
final int index = 0;
// Compiler error. index is immutable
index = 1;
Immutable Objects
Immutable objects cannot be reassigned, just like immutable variables.
// Java code
// Immutable user
final var user = new User();
// Compiler error. user is immutable
user = new User();
This is the same as immutable variables. The difference with immutable objects is that the values inside the object can still change.
// Java code
// Immutable user
final var user = new User();
user.setId(1);
Immutable Classes
Immutable classes are classes that have immutable variables. Immutable classes do not have methods to modify their state. All variables are assigned a value when they are constructed.
// Java code
public class User {
private final long id;
private final String name;
public User(
final long id,
final String name) {
this.id = id;
this.name = name;
}
public long id() {
return id;
}
public String name() {
return name;
}
}
final var user = new User(1L, "name");
Immutable Collections
The way an immutable collection works will depend on the collection's implementation. Immutable collections are implemented one of two ways. The first implementation is when you try to modify the collection, such as adding or removing an item, an exception will be thrown. This shows adding the number 4 to a Java immutable collection.
// Java code
// throws UnsupportedOperationException
immutableCollection.add(4);
The second implementation will allow these operations but return a new collection with the modified items, never modifying the original collection. In the Scala programming language, you add an item to a collection with :+. The add method returns a List.
// Scala code
val list1 = List(1, 2, 3)
// Add the number 4
val list2 = list1 :+ 4
This passes the value 4 to the add (:+) method on list1. The add method constructs a new list with the items of list1 plus the value 4 and assigns it to list2, leaving list1 unmodified. The values of list1 will be [1,2,3] and the values of list2 will be [1,2,3,4].
Conclusion
Mutable variables are variables that can be reassigned a value, while immutable variables cannot be reassigned. Immutable collections will vary depending on the implementation. Try to start with an immutable variable and then change it to mutable if it needs to change.