Nested Classes in Java
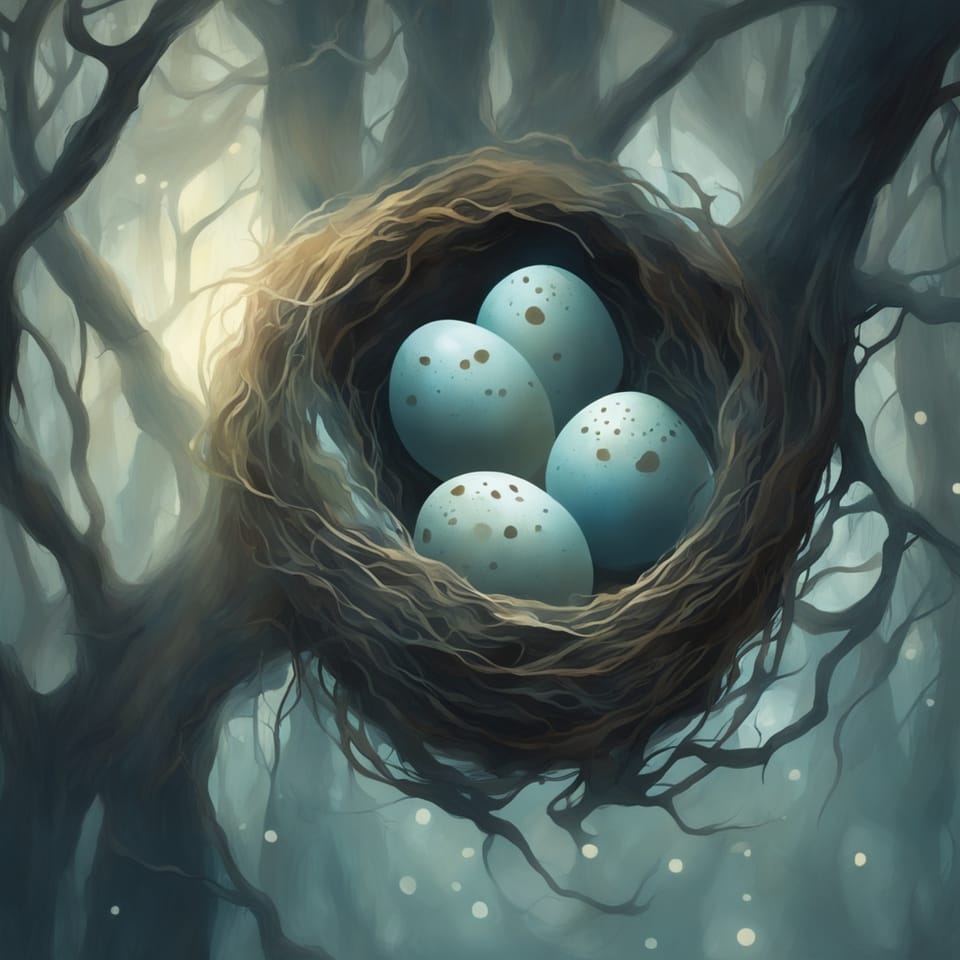
Nested Classes
Nested classes are static classes declared inside of another class. Nested classes are just like normal class and can define variables, methods, constructors, etc. Even though they are declared inside the same file as another class, they are completely independent of the outer class, as though they were defined in a separate file. Unlike an inner class, they cannot access the methods and variables in the outer class. Inner classes can be marked with any access modifier. You can define a nested class with the following:
public class OuterClass {
public static class NestedClass {
public static final int CONSTANT = 11;
private int x = 10;
public void method() {
// ...
}
}
}
This defines an outer class called OuterClass and a nested class called NestedClass.
Instantiating Nested Classes
Nested classes are instantiated the same way as any other class. Using the example above, if you are inside the class it is defined in, you can create an instance with the following:
public class OuterClass {
public void method() {
final NestedClass nestedClass = new NestedClass();
}
// ...
}
If you are outside the class it is defined in, you can create an instance with the following:
public class AnotherClass {
private final OuterClass.NestedClass nestedClass =
new OuterClass.NestedClass();
}
Accessing Static Variables and Methods
Accessing static variables and methods in nested classes will change once again depending on if you are inside the class it is declared in or not. This section will use the following example:
public class OuterClass {
public static class NestedClass {
public static final String GREETING = "Hello";
public static void method() {
}
}
}
If you are inside the outer class, you can access static variables and methods just like any other variable or method.
NestedClass.GREETING;
NestedClass.method();
If you are outside of the outer class, you access static variables and methods with the following:
OuterClass.NestedClass.GREETING;
OuterClass.NestedClass.method();
Conclusion
Nested classes are static classes defined inside another class. Nested classes allow you to encapsulate the class and protect who can create instances of it.