Nested vs Inner Classes in Java
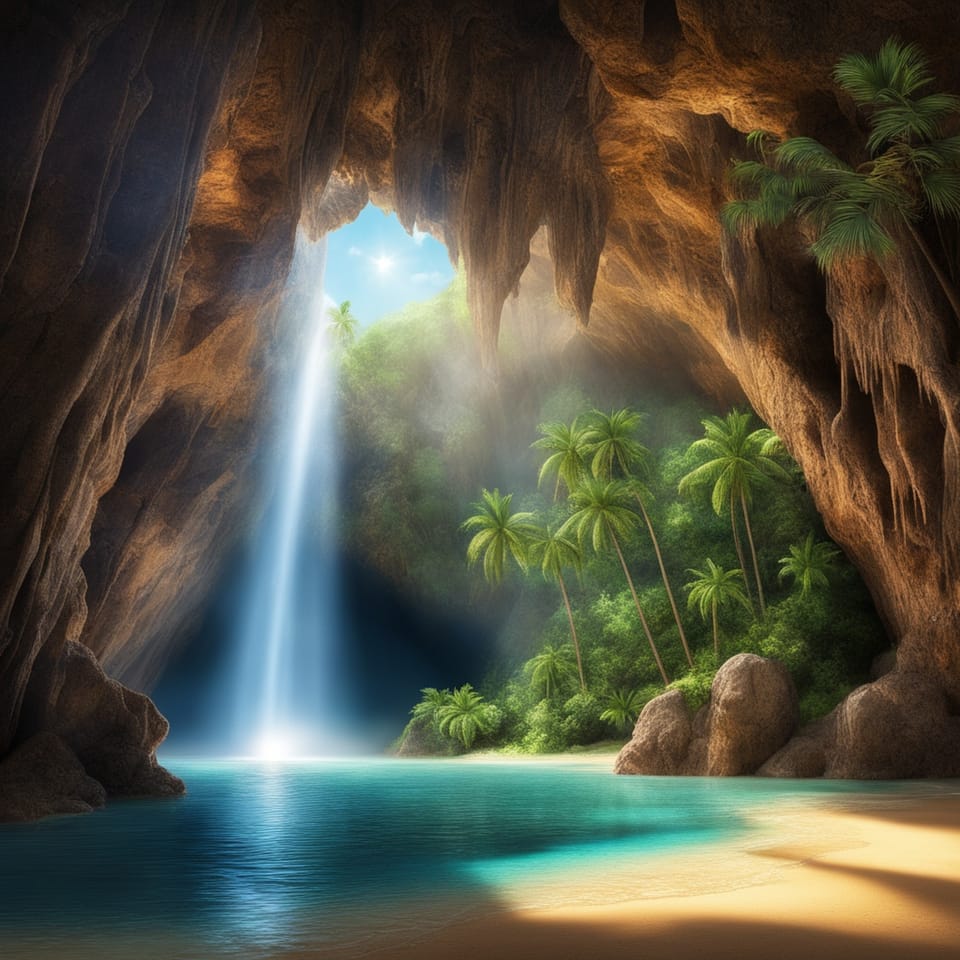
Outer Classes
An outer class is the class that is declared in a Java file that matches both the class name and the Java file name.
// OuterClass.java
public class OuterClass {
}
This is usually what you are working with when working with classes.
Nested Classes
Nested classes are static classes defined inside another class.
public class Customer {
public static class Account {
}
}
A nested class is a completely independent class, even though they are defined inside of another class. They cannot access variables or methods in the outer class. In terms of what the Account class can access in the example above, it wouldn't be any different than defining them in separate files.
// Java code
// Customer.java
public class Customer {
}
// Account.java
public class Account {
}
Inner Classes
Inner classes, sometimes called non-static nested classes, are classes defined inside another class just like nested classes. An inner class is a class that has access to the outer classes variables and methods. Outer classes also have access to an inner classes methods and variables, even if they are marked as private.
public class OuterClass {
private int x = 3;
public void innerExample() {
final var inner = new InnerClass();
inner.y = 10;
}
public class InnerClass {
private int y = 8;
public void print() {
System.out.println(x);
}
}
}
This shows an example of accessing the inner classes variable y in the outer class and accessing the outer classes variable x inside the inner class. This same thing can be done with methods in both the inner and outer classes.
When Should You Use Nested and Inner Classes?
Nested and inner classes are useful when you have a class that is only used inside of another class. This allows you to encapsulate the nested or inner class inside the outer class. If a nested or inner class constructor is marked as anything but private, it is probably better to have that class in its own file instead of being an inner class.
If you are using nested or inner classes to encapsulate data in a class, a nested record class is probably the better use case. Nested record classes follow the same rules as nested classes.
Conclusion
Nested classes and inner classes are both classes that are declared inside of another class. They are both used for encapsulating behavior. Inner classes can share variables and methods of the outer class, but nested classes can't.