Number Operators in Swift
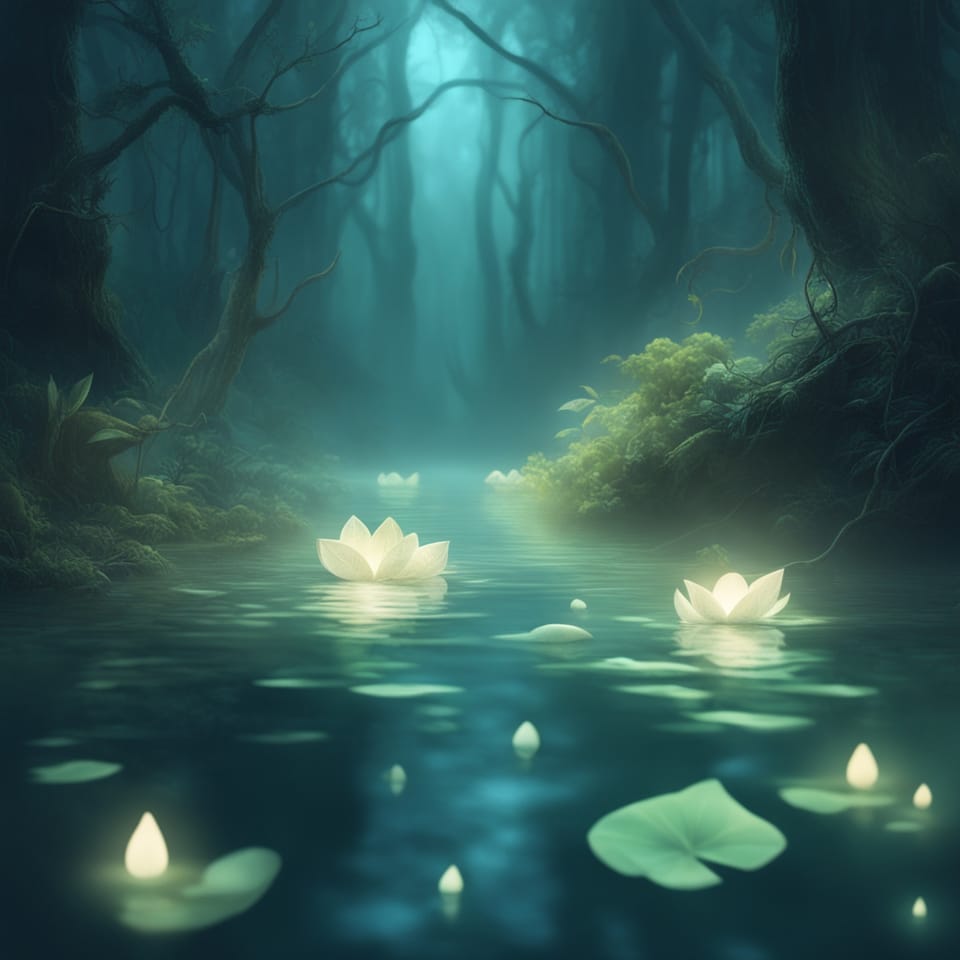
Number Operators
Number operators allow you to do math in code. This can be done with literal values or with variable values. Operators have an order or operations just like they do in math. If you need to control the order of operations, this can be done with parentheses.
Addition Operator
Addition is done using the + operator. This is used to add one number to another.
print(1 + 1) // 2
Subtraction Operator
Subtraction is done using the - operator. This is used to subtract a number from another number.
print(2 - 1) // 1
Multiplication Operator
Multiplication is done using the * operator. This is used to multiply two numbers.
print(2 * 3) // 6
Division Operator
Division is done using the / operator. This is used to divide a number by another number.
print(10 / 3) // 3
When working with division, you only get how many times the number is divided by another number. If you need the remainder, you use the remainder operator.
Remainder Operator
The remainder operator is done using the % operator. This operator is also referred to as the modulus operator and is used to find the remainder of a division operation.
print(10 % 3) // 1
Number Assignment Operators
Variables are assigned a value using =.
var x = 1
// x = 4
x = x + 3
Number assignment operators are used to provide a shorthand assignment to a variable. Using assignment operators, this can be shortened with the following:
var x = 1
// x = 4
x += 3
This can be used with any of the operators shown above.
Incrementing and Decrementing
You can increment and decrement number variables with the following.
var x = 0
// increment by one
x = x + 1
// decrement by one
x = x - 1
There is a shorter version of this you can use, and it is supported by any of the operators above.
var x = 0
// increment by one
x += 1
// decrement by one
x -= 1
Whitespace
When using number operators, whitespace is an all-or-nothing. You can either provide whitespace or not, but you can't do both. If you try to have a hybrid approach, it will result in a compiler error.
// This is okay
print(1 + 1)
// This is okay
print(1+1)
// Compiler error
// '+' is not a postfix unary operator
print(1+ 1)
// Compiler error
// Expected ',' separator
print(1 +1)
Conclusion
Number operators allow you to do math on values in code. They have an order of operations similar to math. When using number operators, be mindful of whitespace. Either provide it or not.