Number Systems in Java
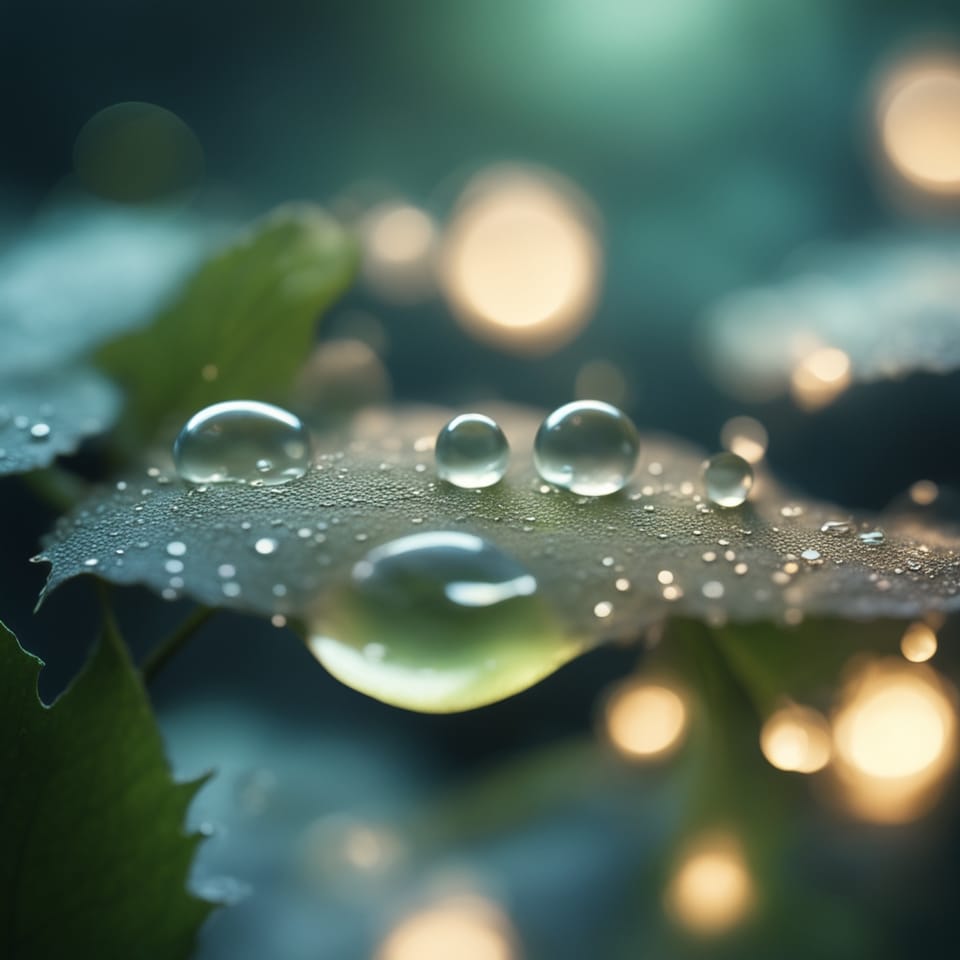
Integer Number Systems
Integer literals can be written using decimal numbers, hexadecimal, octal, and binary.
Decimal
Decimal is a base-10 number system consisting of 0-9 and is what is typically used. These are written just as a number.
final int decimal = 12;
Hexadecimal
Hexadecimal is a base-16 number system consisting of numbers 0-9 and letters A-F. Hexadecimal values are written prefixed with 0x.
final int hex = 0x57;
Octal
Octal is a base-8 number system using numbers 0-7. Octal numbers are written prefixed with a 0.
final int octal = 0325;
Binary
Binary is base 2 and has numbers 0 and 1. Binary numbers are prefixed with 0b.
final int binary = 0b1010111;
Converting Between Different Number Systems
Java supports converting decimal numbers to binary, octal, and hexadecimal. Also, you can convert binary, octal, and hexadecimal back to decimal numbers.
Converting From Decimal
The Integer class has methods on it for converting a decimal number to binary, octal, or hexadecimal.
final int x = 10;
// 1010
final String binaryNumber = Integer.toBinaryString(x);
// 12
final String octalNumber = Integer.toOctalString(x);
// a
final String hexNumber = Integer.toHexString(x);
Converting to Decimal
To convert binary, octal, or hexadecimal to a decimal number, use the parseInt() method on the Integer class. This method takes two parameters, a string value of the number and the radix. The radix is the base in the mathematical numeral system.
final String binary = "1010";
final String octal = "12"
final String hex = "a";
final int binaryAsDecimal = Integer.parseInt(binary, 2); // 10
final int octalAsDecimal = Integer.parseInt(octal, 8); // 10
final int hexAsDecimal = Integer.parseInt(hex, 16); // 10
Passing in the wrong numeral system along with the wrong radix will result in a NumberFormatException.
Conclusion
Java supports decimal numbers, hexadecimal, octal, and binary numbers. Use the Integer class to convert to and from these different systems.