Optionals in Swift
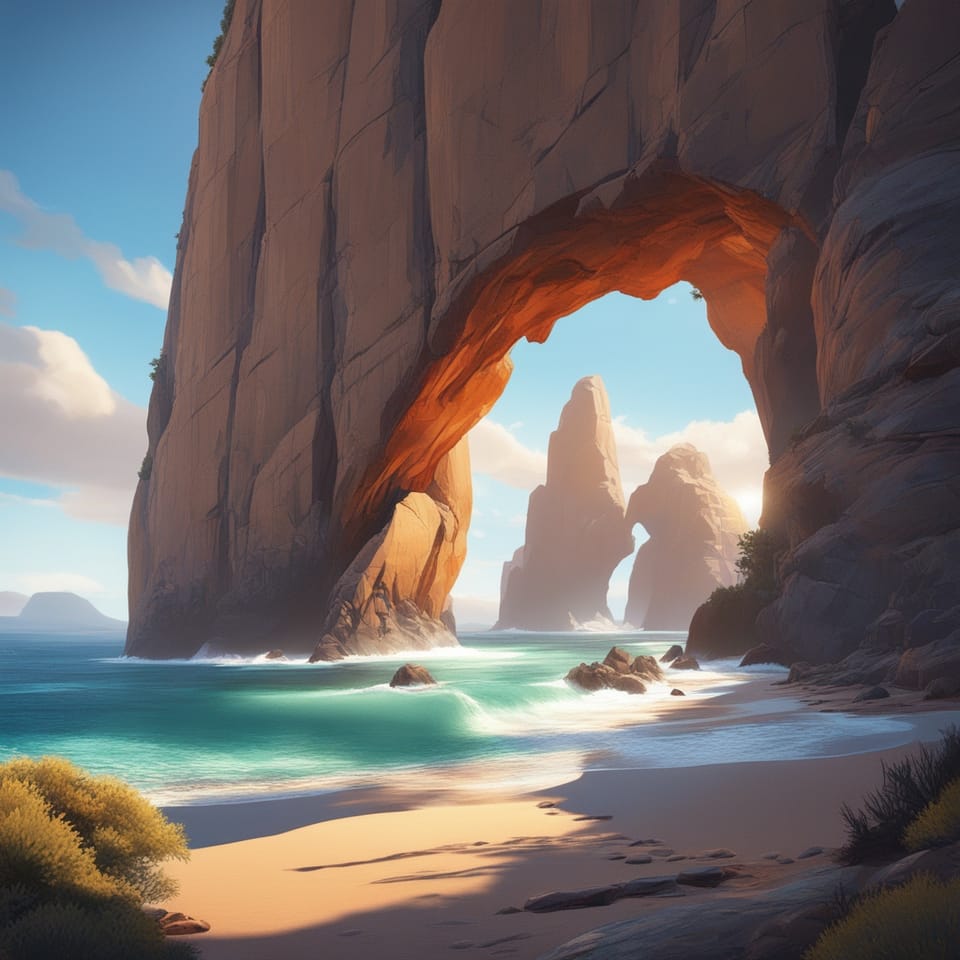
Option
A variable can be assigned a value or not have a value at all. In other languages, a variable not assigned a value is commonly represented as null. Swift has this same concept, but instead of using null, it uses an optional type. An optional type is a value that may or may not have a value assigned to it. It can then be unwrapped to access the value.
Any type can be an optional type. To make it an optional type, the type ends with a ?.
let optional: String? = "value"
This defines an optional String type that has a value. For the absence of a value, you use the keyword nil.
let optional: String? = nil
This is an optional variable that doesn't contain a value. If a variable is defined with the var keyword and there isn't a value set, it is automatically set to nil.
// nil
var optional: String?
If you are coming from a language that uses nulls, this may look like what is happening here, but it isn't the same. The syntax is similar, but a nil value is an optional data type that doesn't have a value. This is nice because you can assign values like you are working with null, but everything is done using an optional and enforced by the compiler.
Optional Binding
Similar to checking for null in other languages, you can check for an empty optional with the following:
if optional == nil {
print("Optional is empty")
} else {
print("Optional has a value")
}
This is such a common thing that you can actually bind an optional value to a variable in an if/else statement. This allows you to define a variable for the optional value if it isn't nil.
if let actualValue = optional {
print("Optional value is \(actualValue)")
} else {
print("Optional is empty")
}
This declares a variable called actualValue and assigns it the value of optional if optional is not nil. If it is nil, the else statement will be executed instead.
You can bind multiple values in the same if statement.
if let actualValue1 = optional1, let actualValue2 = optional2 {
// ...
}
You can also use these variables to have further logic in the if statement.
if let actualValue1 = optional1,
let actualValue2 = optional2,
actualValue1 == actualValue2 {
// ...
}
Nil-coalescing Operator (??)
Using optional bindings like what was shown in the previous example is nice for more complex code, but for more similar code, there is a better optional. Similar to a ternary, the nil-coalescing operator allows you to do something similar.
let actualValue = optional ?? "Empty value"
If optional is nil, it will use the value "Empty value" and if it isn't, it will unwrap the optional and use that value instead.
Implicit Wrapping Optionals
Non-optional values will be implicitly wrapped as an optional. This happens when assigning a non-optional to an optional variable.
let value1: String = "value"
let value2: String? = value1
This same thing is done with method parameters.
func print(_ message: String?) {
}
Even though this method has an optional parameter, you can pass both an optional and a non-optional value to it. If the value isn't an optional, it will be implicitly converted to an optional when passed as an argument to the method.
let optional: String? = nil
let nonOptional: String = "value"
print(optional)
print(nonOptional)
Implicitly Unwrapping Optionals
You can implicitly unwrap an optional using the ! operator. This is written after the variable name.
let optional: String? = "value"
let nonOptional = optional!
The variable nonOptional will be assigned the value "value" and not be an optional. This should only be used when it is obvious that the optional is going to have a value. Using this operator on nil will result in a fatal error.
// Fatal error: Unexpectedly found nil while unwrapping an Optional value
print(nilOptional!)
Conclusion
Optionals are Swift's way to handle variables that can have a value or not have a value. Optionals that don't have a value are nil. There are multiple ways you can wrap and unwrap optionals that make them very natural and easy to use.