Ranges in Swift
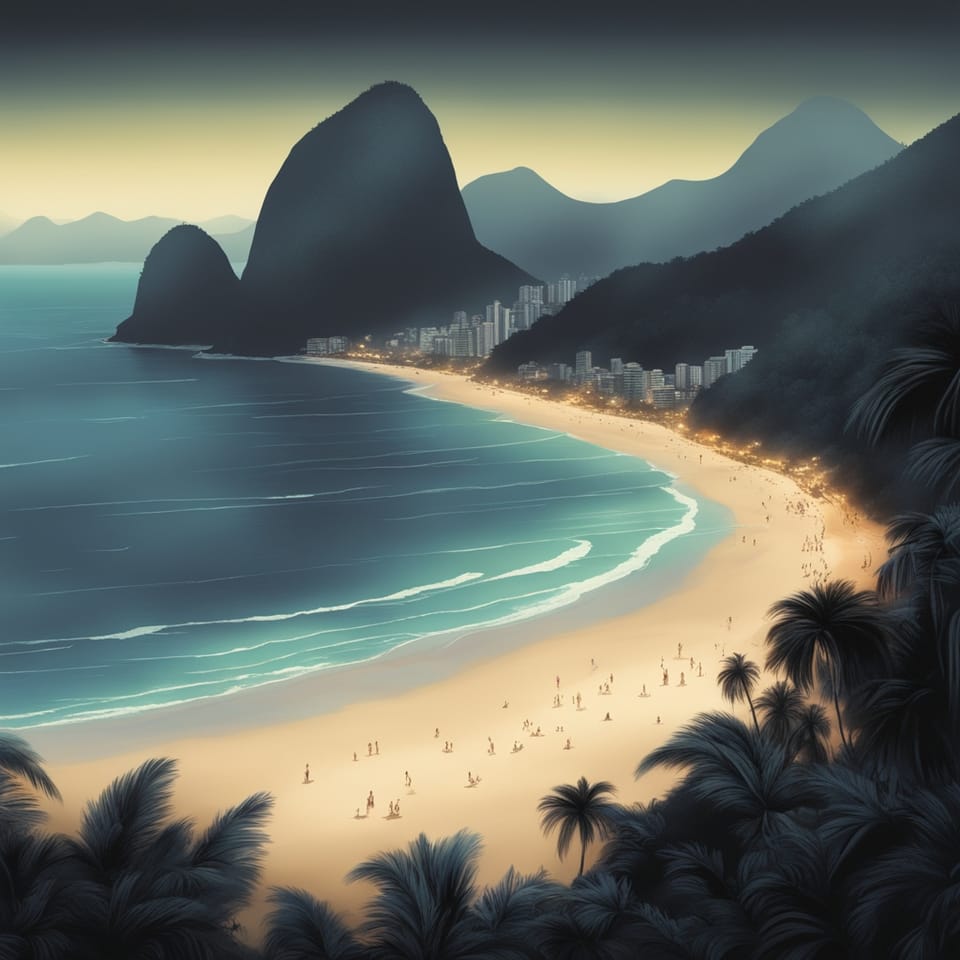
What Are Ranges?
Ranges are a way to represent a sequence of values within a specific range. Ranges are defined with a starting and ending value. A range is similar to the following Array:
let numbers = [1, 2, 3, 4, 5]
Closed Ranges
A closed range is a range that starts with a value and ends with a value. The starting and ending values will be included, as well as all other values in between. A closed range can be written with the following:
let range = 1...5
You can use ranges in for loops to iterate over the range.
for value in 1...5 {
print(value)
}
This would produce the following output:
1
2
3
4
5
The starting number in a closed range must be less than or equal to the ending value. If it isn't, this will result in a fatal error.
// Fatal error: Range requires lowerBound <= upperBound
let range = 2...1
Half-open Range
Half-open ranges are the same as closed ranges except they don't include the ending value. They are written a little differently than a closed range.
for value in 1..<5 {
print(value)
}
This will produce the following output:
1
2
3
4
Unbound Range
Unbound ranges are where you do not provide either a starting value or an ending value. In a loop, you could create an infinite loop using an unbound range with no ending value.
for value in 1... {
if value == 5 {
break
}
print(value)
}
Since there is an if statement with a break in it, it will break out when the value is 5, so the program won't crash. This will produce the following output:
1
2
3
4
You can also use these types of ranges in an Array. The ending number will become the length of the array. This allows you to do some interesting things with the Array and start at a specific index, which would be the starting value.
let numbers = [1, 2, 3, 4, 5]
print(numbers[2...])
This will start printing each of the numbers starting at 2. This will produce the following output:
[3, 4, 5]
You can also provide an ending value instead of a starting value. This will start at the beginning and end once the ending value is met.
let numbers = [1, 2, 3, 4, 5]
print(numbers[...3])
This will produce the following output:
[1, 2, 3, 4]
If the ending value is larger than the array size, it will result in a fatal error.
let numbers = [1, 2, 3, 4, 5]
// Fatal error: Array index is out of range
print(numbers[...10])
Ranges and Methods
A range produces an object. Depending on what type of range you are using, it will change what type of object it returns. Each type is shown for each different range in the following:
let closedRange: ClosedRange<Int> = 1...5
let range: Range<Int> = 1..<5
let rangeThrough: PartialRangeThrough<Int> = ...5
let rangeFrom: PartialRangeFrom<Int> = 1...
Since a range returns an object, they can be passed to methods as arguments. The Int.random() method takes a ClosedRange as a parameter.
let randomNumber = Int.random(in: 1...5)
Conclusion
Ranges are a way to represent a sequence of a range of values. There are multiple different types of ranges that you can use. The most common one is the closed range. Ranges produce an object so they can be used in things like an Array and as method arguments.