Repeat-while Loops in Swift
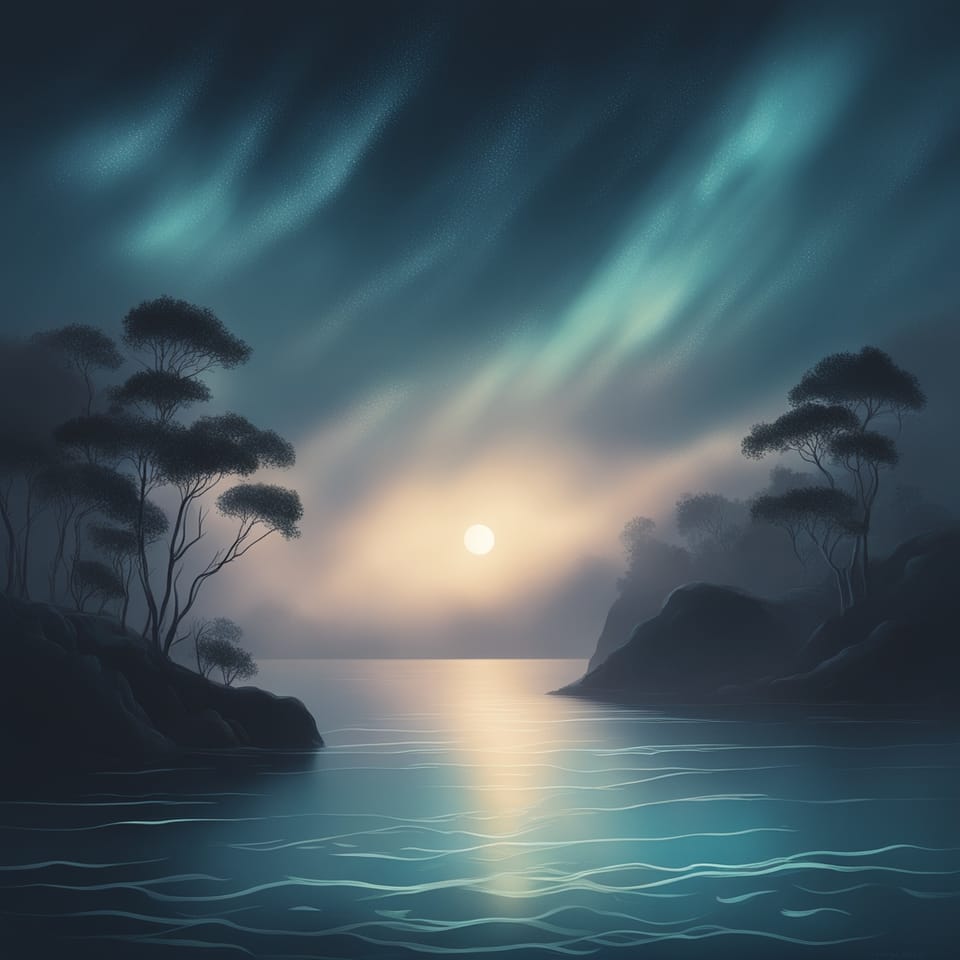
What Are Repeat-while Loops?
A repeat-while loop will always execute an iteration of the loop. After it executes an iteration of the loop, it will then evaluate a condition to see if that condition is true. If it is true, it will execute an additional iteration of the loop. If it is false, it will not execute another iteration of a loop.
Repeat-while loops are the same as while loops except they evaluate the condition at the end of the loop instead of the beginning of the loop. If you are familiar with C-like languages, this same thing is done using a do-while loop.
Repeat While Loop Syntax
A repeat while loop is similar to a while loop but instead starts with the repeat keyword instead of the while keyword.
var counter = 0
repeat {
print(counter)
counter += 1
} while counter != 5
This first executes the block of code in the repeat block. It then evaluates the condition in the while clause to see if it is true. If it is true, it will execute another iteration of the loop. If it is false, it will not execute any more iterations of the loop. This will produce the following output:
0
1
2
3
4
Repeat-while loops are good to use when you have an unknown number of iterations but need to always execute at least one of those iterations.
Conclusion
Repeat-while loop guarantees that at least one iteration of a loop will be executed. They execute the same as a while loop with the exception of evaluating the condition at the end of the loop. In C-like languages they are done using do-while loops.