Scope and Good Variable Naming
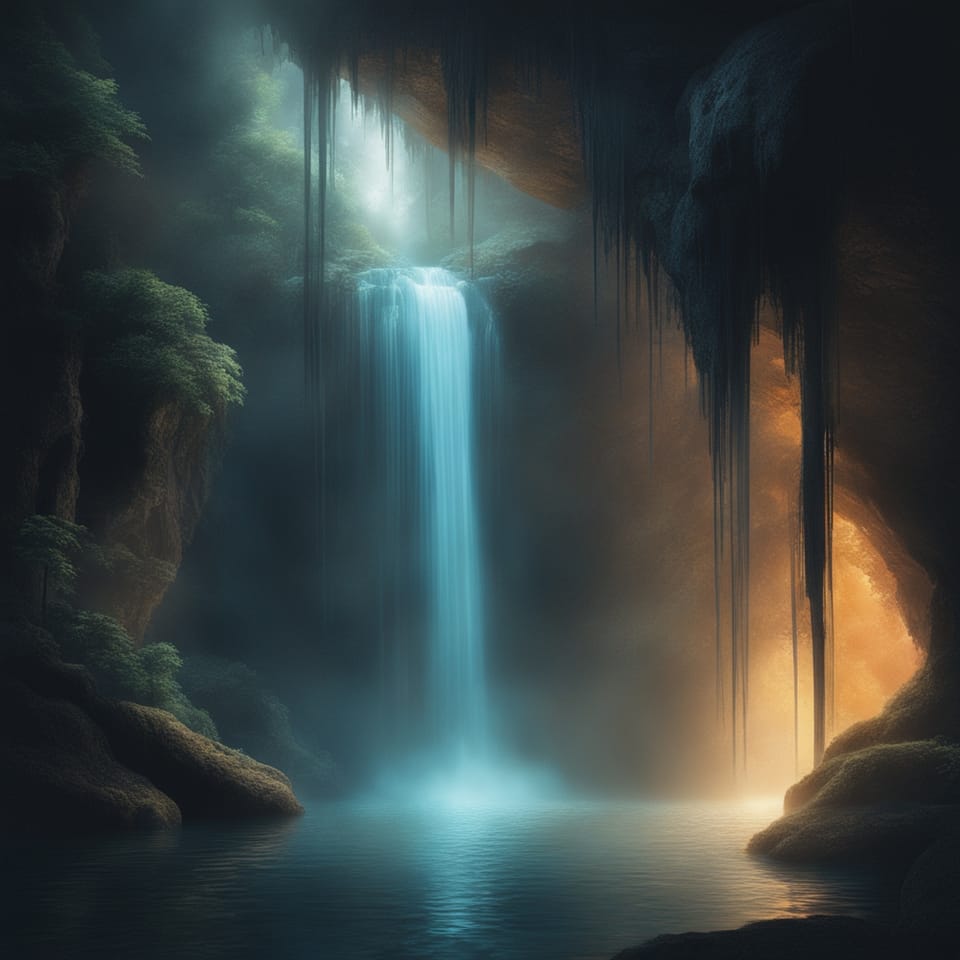
Good Variable Naming
There are a lot of great resources on good variable naming. This article is going to assume you are aware of good variable naming practices and take a different approach focusing on the scope of a variable and how you should name it. First of all, all good variable naming practices should always be considered, but you can make variable names even better when considering the scope. The scope of the variable is really the context that it should be named in.
Constants
When naming global constants, it is all about what information needs to be in the name. With such a large scope, longer and more descriptive names are okay, and abbreviated names should be avoided. If there isn't context, you will need to add that in the name as well.
# Python code
# Global constant
DEFAULT_MESSAGE_SIZE_IN_BYTES = 1024
This shows a global constant in Python. There isn't any context, so the context has to be added into the name. If this variable name had anything removed from it, it would cause confusion. Even though this is a long name, it is clear what it is. In a language such as Java, everything has to be in a class. This allows you to have context associated with the constant. Because of this, you can eliminate the context in the name and reduce the length of the name.
// Java Code
public class Message {
// Global constant
public static final int DEFAULT_SIZE_IN_BYTES = 1024;
}
When this constant is accessed, it would look like the following:
// Java code
Message.DEFAULT_SIZE_IN_BYTES
Private constants don't have to be as descriptive and have context associated with them. Depending on the code and the context in which the constant is used, you may be able to get away with a name of DEFAULT_SIZE.
// Java code
public class Message {
private static final int DEFAULT_SIZE = 1024;
}
Instance Variables
Instance variables, also called member variables, are the easiest and most natural to name. Instance variables are the state inside the object. Taking any real world example, it is easy to see the properties of an object. A Pet class may have a type, color, and name. A Person class may have a name, address, and age. A Product may have a name, description, price, etc.
Instance variables should be named to describe the state that variable stores and usually start with a noun. They should describe the value they hold and no more.
// Java code
public class Address {
private String street;
private String city;
private String state;
private String zip;
// ...
}
Adding anything to these instance variables wouldn't add any value and only complicate the code. They already have context of an address, so that doesn't need to be added to the names.
Don't oversimplify instance variables. In a Product class, you could have a name variable. In a Person class, you may have a name variable too, but is this a full name, a user name, or something else? In a Person class, being more descriptive, you could name the variable fullName instead.
Instance variables shouldn't be prefixed or postfixed with anything unless it is a standard in the language you are working in.
Local Variables
Local variables should be short names and describe the intent of their usage. If you were writing a test to test a query, the variables in your dataset might have the following names.
// Java code
final User toRetain;
final User toDelete;
final User toFind;
This same thing applies to function and method parameters.
// Java code
public String addPadding(final String rawValue) {
final String paddedValue;
// ...
}
Although they are short in scope, don't abbreviate names unless the abbreviation is more common than the full name. An example of this would be id instead of identifier.
Conclusion
Instead of making all variables equal in terms of how you name them, consider the scope of the variable. Good variable naming practices should always be used, but considering the scope of the variable can help you better name them.