Simple Return Statements and Values
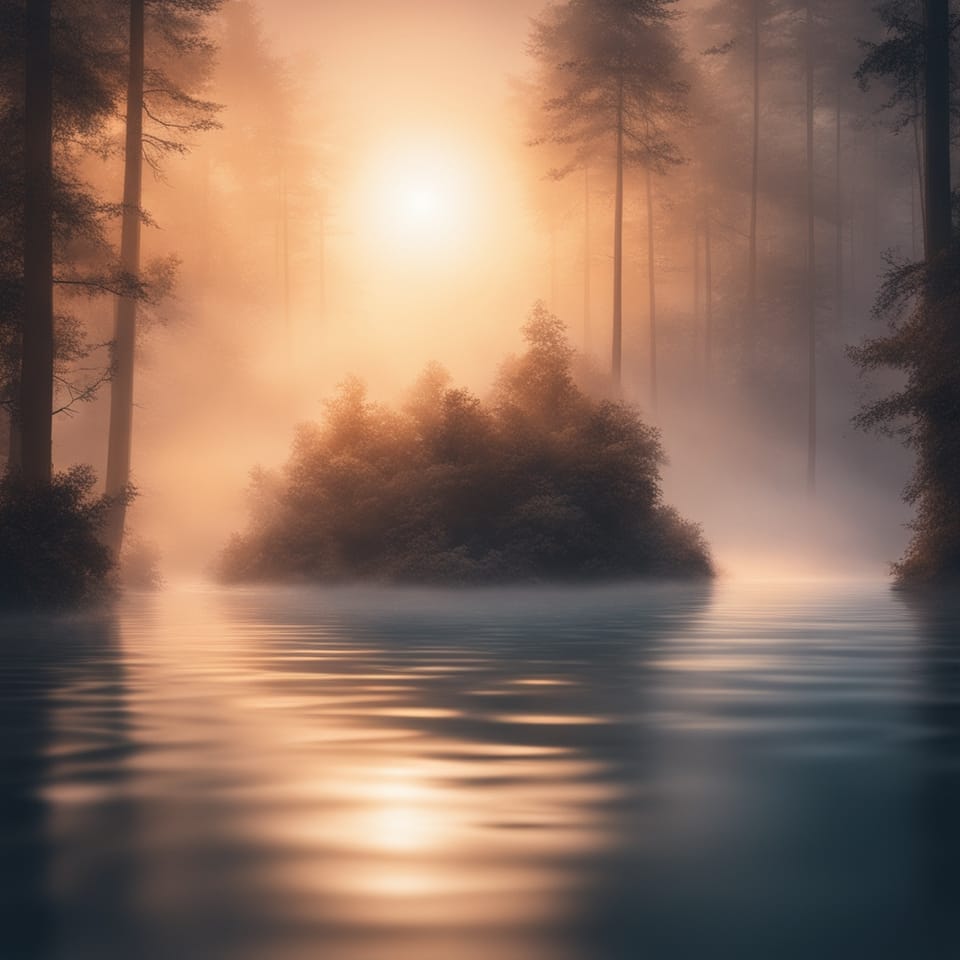
When Should You Declare the Variable Being Returned?
One standard is to declare the variable you are returning at the top of the method or function. This is a great standard to implement if the variable can also be assigned a value. For example, if you have a factory method that is declaring an object, populating it, and then returning it, this is perfect.
If you can't assign the variable a value, it is better to declare the variable when you can both declare and assign it a value. Variables that are declared but not assigned add complexity to the code.
Naming
Some teams will standardize on the name of the variable returned, making it uniform across the code base. For example, retVal is a common name that is used.
// Java code
public Customer create() {
final var retVal = new Customer();
retVal.setId(UUID.randomUUID());
return retVal;
}
Using retVal for the name isn't the best. It is both an abbreviation and is hard to type. A simpler name would be result.
// Java code
public Customer create() {
final var result = new Customer();
result.setId(UUID.randomUUID());
return result;
}
This is short and easy to type.
It is common, especially when working with an object, that the object's name will be used for the variable name. This isn't bad either and can make the code more clear when using type inference.
// Java code
public Customer create() {
final var customer = new Customer();
customer.setId(UUID.randomUUID());
return customer;
}
Don't Be Redundant
Don't declare and assign a value to a variable, then immediately return it like the following:
// Java code
public int add(final int num1, final int num2) {
final var result = num1 + num2;
return result;
}
This is redundant and makes it more complex than it needs to be. Keep it simple.
// Java code
public int add(final int num1, final int num2) {
return num1 + num2;
}
Conclusion
There really isn't a whole lot to do with return statements. Come up with a standard that works for your team for return statements and values. Standardize on variable declarations and don't be redundant. This will help make your code more uniform across your code base.