Simplifying Throws Clauses in Java Unit Tests
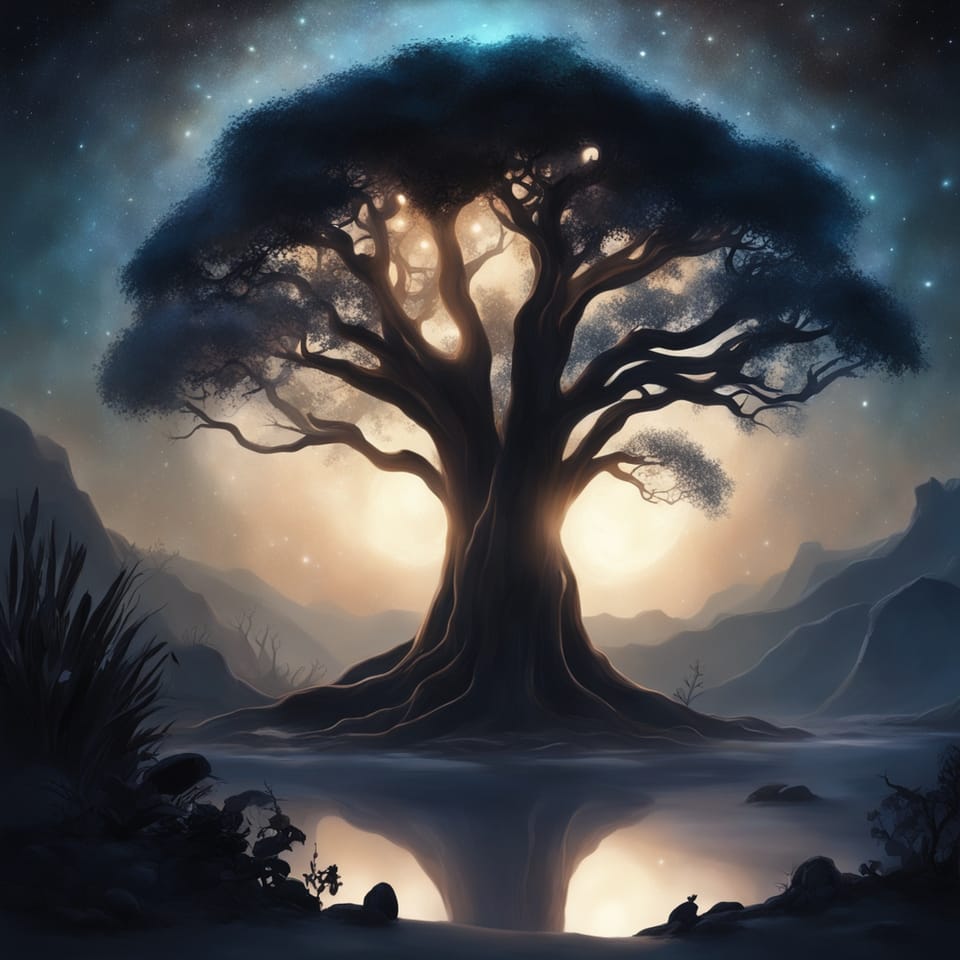
Checked Exceptions
In production code, you may have a method signature such as the following:
public String findName(final Uuid id)
throws DaoObjectNotFoundException, DaoConstraintException
When it comes time to write a test for this method, what will the throws clause on the test method signature look like?
@Test
public void findsName() throws DaoObjectNotFoundException, DaoConstraintException {
}
Down the road, if an additional checked exception is added to this method, you will then have to add it to the throws clause for each unit test that tests this method.
Don't List Your Exceptions
Think of a unit test as a main() method. Main methods don't make sense to have anything but Exception in the throws clause.
public static void main(final String[] args) throws Exception {
}
This same train of thought should be applied to unit tests. Listing each checked exception in the throws clause doesn't make sense in unit tests and only causes headaches.
If a method in a test throws one or more checked exceptions, always just throw Exception.
@Test
public void findsName() throws Exception {
}
Refactoring
Listing each checked exception in a unit test's throws clause can make refactoring a nightmare. You only care if an exception is thrown or not in a test. Listing them in the throws clause doesn't matter. It either has a throws clause with only Exception or it doesn't have a throws clause.
Source Control
Listing each checked exception in the test method's throws clause only clutters the changes in source control. This will show an additional change for each signature changed. This is something that you aren't going to be concerned with and will only slow you down.
Conclusion
Since you can't silence checked exceptions, make your unit tests resilient to changes by only using Exception in your throws clause. Keep your source control clean and don't waste your time thinking about a method's throws clause in unit tests.