Statement vs Expression
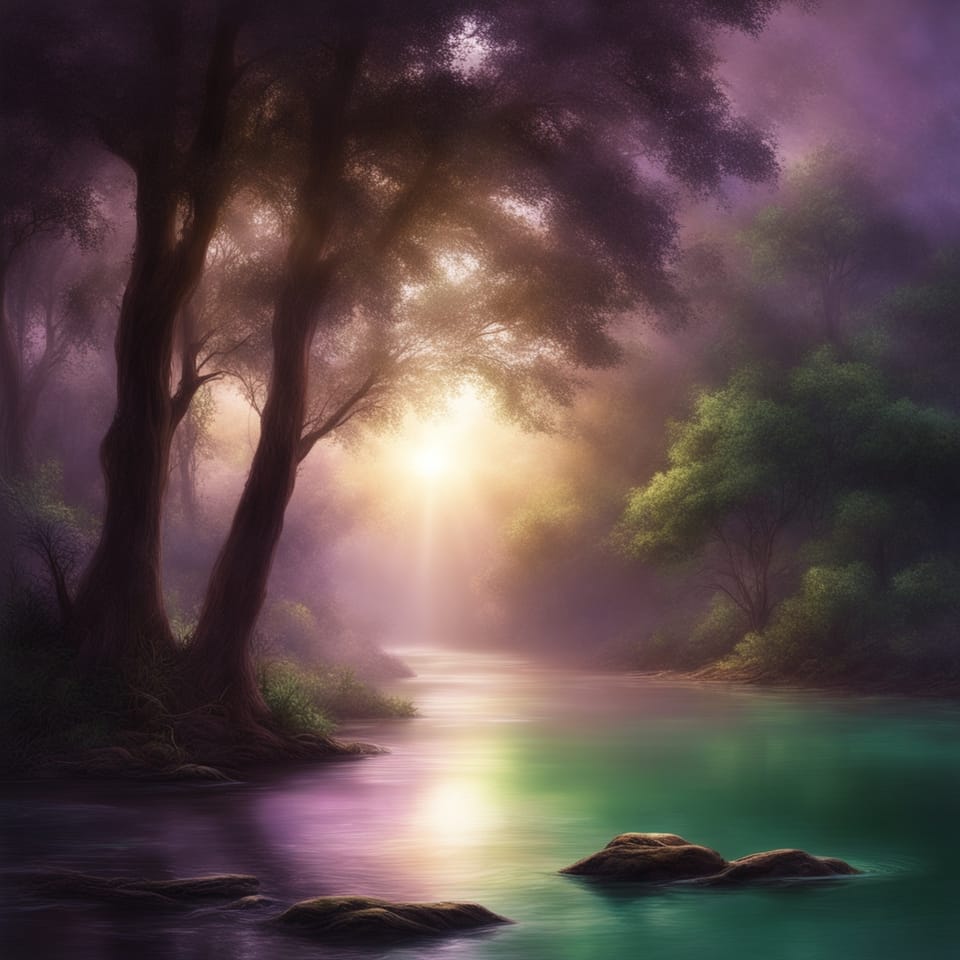
The differences between statements and expressions are obvious in some cases, but others can be kind of confusing, especially in the case of control flow. If statements and if expressions are both used for control flow, what is the difference?
What Are Statements?
Statements execute some type of action. Statements can have side effects, such as modifying some type of state or printing something. For example, the following statement would print something out to the console.
// Java code
System.out.println("Hello");
Statements are used to set a value on an object.
// Java code
user.setId(123);
Statements can come in the form of control flow. For example, using an if statement.
// Java code
if (i == 0) {
// ...
} else {
// ...
}
What Are Expressions?
An expression evaluates something and returns a value. Expressions usually don't have side effects. An example of an expression would be.
// Java code
(i > 0)
This expression would return a boolean. You could return an integer with the following:
// Java code
(7 + 8)
Expressions are used for the boolean evaluation of an if statement.
// Java code
if (i > 0) {
// ...
}
Control Flow Expressions
In some languages, you have control flow expressions instead of control flow statements. This means your control flow expressions return a value. For example, in the Scala programming language, you have if expressions.
// Scala code
val result = if (i > 0) {
"Selected"
} else {
"Not Selected"
}
This assigns a value to the variable result based on the evaluation of the if expression.
Expressions such as the if expression can be used like if statements by just ignoring what is returned.
Loops can even be expressions in some languages. You can use a for loop, for example, in the Scala programming language, to loop through a collection and return a new collection.
// Scala code
val numbers = for (n <- List(1, 2, 3)) {
yield n * 2
}
The variable numbers will be a new collection with the numbers 2, 4, and 6.
Pure Functions
The behavior of a pure function aligns with the definition of an expression. A pure function always returns the same output when it is given the same input. Given the following expression:
// Java code
(1 + 2)
This expression could be written using a pure function.
// Java code
public int add(final int num1, final int num2) {
return num1 + num2;
}
add(1, 2);
Conclusion
Statements are used to execute an action. Expressions return something. Statements can have side effects, while expressions usually don't.