Static Methods in Java
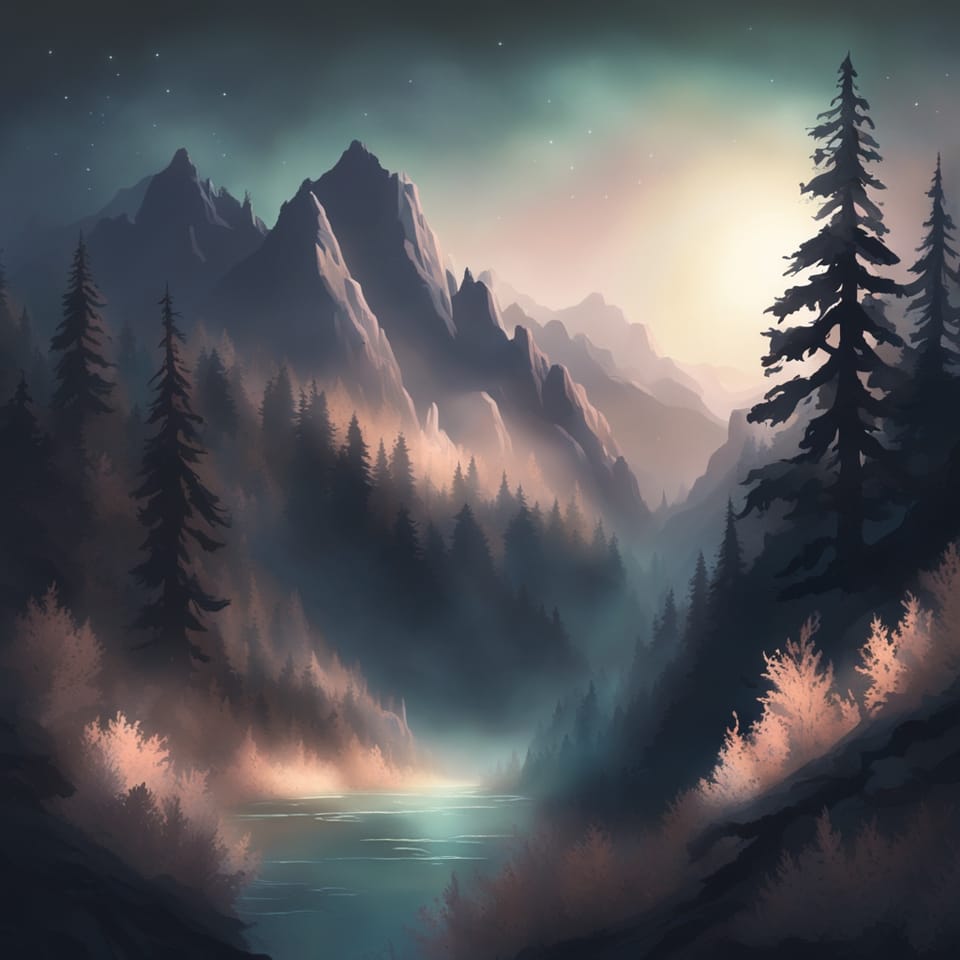
Class Static Methods
Static methods are methods marked with the static keyword. Static methods, like static variables, only have one copy. They cannot access instance variables or instance methods. They can access class variables, constants, and static methods. Also, instance methods can access static methods. Static methods are useful for methods that don't require state such as pure functions or modifying static variable state.
public class MyClass {
// Instance method
public void sayHello() {
print("Hello World");
}
// Static method
public static void sayGoodbye() {
print("Goodbye!");
}
// Static method
private static void print(final String text) {
System.out.println(text);
}
}
This example shows accessing static methods in both instance methods and static methods.
If you are outside of the class the static method is defined in, you access it with the following:
MyClass.sayGoodbye();
Interface Static Methods
Interfaces can have static methods defined on them just like a class can. They can be useful for helper methods in default methods or be used in classes that implement that interface. They follow the same rules as static methods in classes.
public interface MyInterface {
public static void print(final String text) {
System.out.println(text);
}
}
If you are inside the interface, you can access the method the same as what was shown in the class that defines a static method. If you aren't in the interface, you have to access it with the following:
MyInterface.print("Hello");
Overloaded Static Methods
Method overloading is when you have multiple methods with the same name but that take different method parameters. Static methods also support method overloading. They follow the same rules as instance methods do with overloading.
public class MyClass {
public static void print(final String text) {
System.out.print(text);
}
public static void print(
final String text,
final Exception e) {
System.out.println(e.getMessage() + ": " + text);
}
}
Conclusion
Static methods are methods that contain only one copy of the method, similar to a static variable. They are accessed the same inside the class they are in as instance methods. If you are outside the class, you access it with the class name followed by the method. Static methods are supported on interfaces and also support method overloading.