String Interpolation in Swift
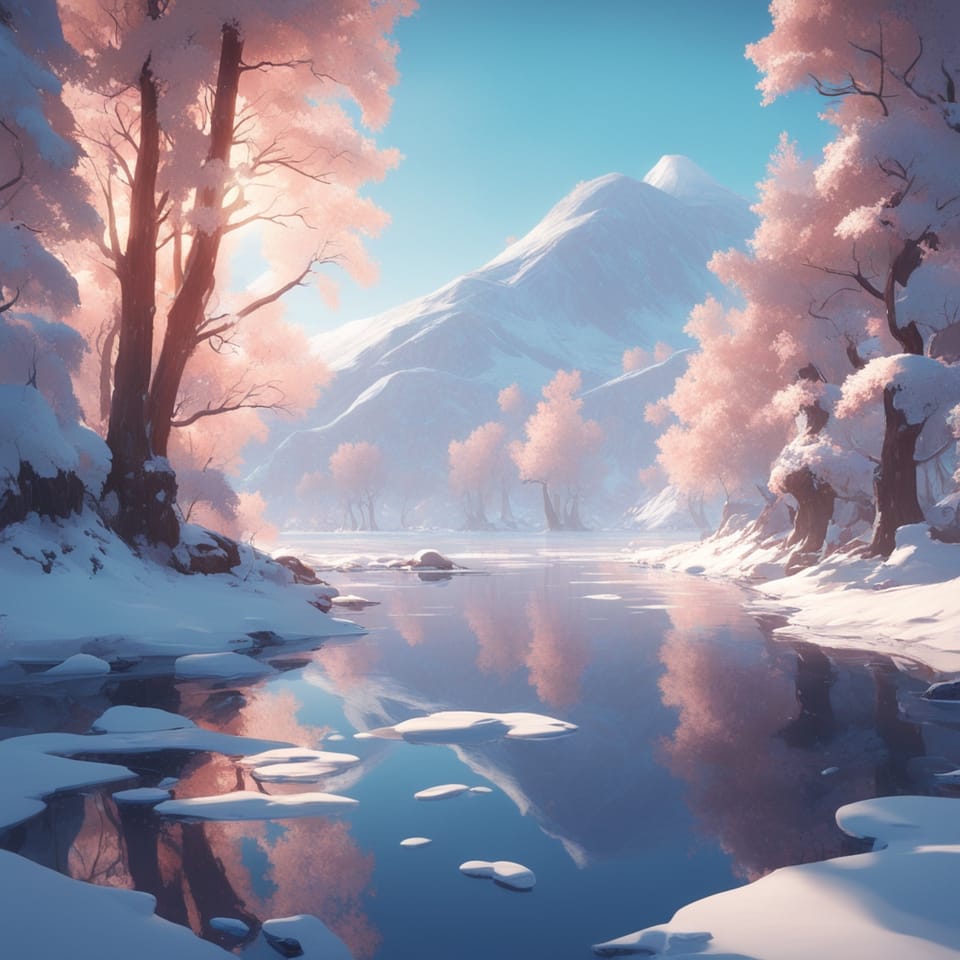
String Concatenation
When building a string in programming, it is common to have to concatenate other values to that string. In any language, this can get ugly quickly. In Swift, it is worse because you have to convert a non-String to a String. It is also problematic. When building something like an error message, it is easy to omit a space when one is needed.
let total = 109.92
let items = 3
// "Total amount due: $109.92 for 3 items"
print("Total amount due: $" + String(total) + " for " + String(items) + " items")
To be able to cleanly build a String, this is where interpolation comes in.
Interpolation Using Variables
String interpolation allows you to insert values into a String value instead of having to concatenate the String. You also no longer have to convert non-String values to Strings. String interpolation is done using the escape character \ followed by the value you want to insert into the string in parentheses. Using the previous example, it could be rewritten using string interpolation with the following:
let total = 109.92
let items = 3
// "Total amount due: $109.92 for 3 items"
print("Total amount due: $\(total) for \(items) items")
You can easily see the value of the String with the values that will be inserted, preventing any spaces from being omitted unintentionally.
Interpolation Using Expressions
String interpolation can be used with expressions as well. If you need to calculate a value, you can do it in another variable and then insert that variable's value into the String. If that value isn't needed anywhere else other than the String, you should just use an expression in the String instead.
let cost = 39.99
let items: Double = 3
// Your total is: $119.97
print("Your total is: $\(cost * items)")
Conclusion
If you are concatenating two string values together, string concatenation is okay for this. When you get into more complicated scenarios, always use interpolation instead.