Strings in Swift
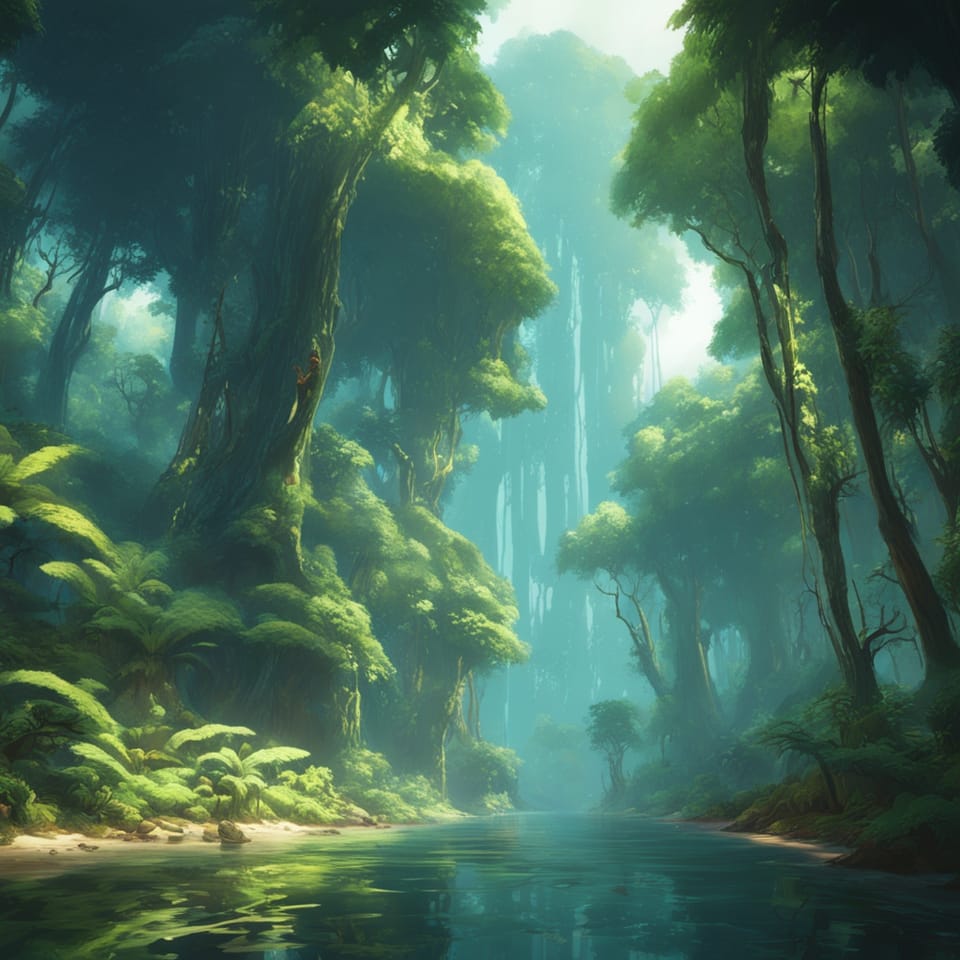
Strings
Strings allow you to have zero or more characters represented as a single value. They can be any supported Unicode character. Strings are written with the following with or without the type annotation:
let greeting = "Hello World"
Special Characters
Special characters, such as a Unicode character or double quotes, must be escaped in a String. Special characters are escaped using a \ before the character.
let doubleQuote = "\""
let tab = "\t"
let lineFeed = "\n"
Unicode characters start with \u followed by the hexadecimal number in braces.
let smiley = "\u{1F600}" // 🙂
String Length
The length of a String can be calculated by using count on a String value. This can be done on either a literal string value or a String variable.
let greeting = "Hello World"
print(greeting.count) // 11
print("goodbye".count) // 7
String Concatenation
Strings can be concatenated using the + operator.
let hello = "Hello"
let world = "World"
let greeting = hello + " " + world
print(greeting) // Hello World
Variables that are declared with the var keyword instead of the let keyword can be appended to using the += operator.
var greeting = "Hello"
greeting += " "
greeting += "World"
print(greeting) // Hello World
If you have a Character you want to append to a String, you can use the append() method. This way, you do not have to cast the Character to a String when concatenating it.
var greeting = "Hello World"
let exclamationMark: Character = "!"
greeting.append(exclamationMark)
String Equality
You can check to see if a String is equal to another String value using the == operator.
let greeting = "Hello World"
print("Hello World" == greeting) // true
print("Goodbye" == greeting) // false
You can also check to see if a String is not equal to another String value using the != operator.
let greeting = "Hello World"
print("Hello World" != greeting) // false
print("Goodbye" != greeting) // true
Unicode
Swift's String data type is built using Unicode scalar values. Each character in a String represents a single extended grapheme cluster. In Unicode, a single character can be represented by one or more code points. For example, the character "a" can be represented by a single code point, U+0061, while the character "é" can be represented by two code points, U+0065 and U+0301. Using a single or multiple scalars on a Character data type is shown using the same character "é" in the following example:
let oneScalar: Character = "\u{00E9}" // é
let twoScalars: Character = "\u{0065}\u{0301}" // e and ́
print(oneScalar) // é
print(twoScalars) // é
Because of this, it is possible that string concatenation will change the String's value but not change the length of the String. This is shown in the following example:
var cafe = "cafe"
print(cafe) // cafe
print(cafe.count) // 4
cafe += "\u{0301}" // ́
print(cafe) // café
print(cafe.count) // 4
Since different characters can require different amounts of memory, operations like count cannot be calculated without iterating over each Unicode scalar in the String. This is something you need to be aware of, especially when working with large String values.
Conclusion
Strings are a data type that allows you to store zero or more characters in a value. You can add to them if they are a mutable String and get the length of the String. Comparison operators can be used to check if two Strings are equal or not equal.