Structure of a Java Source File
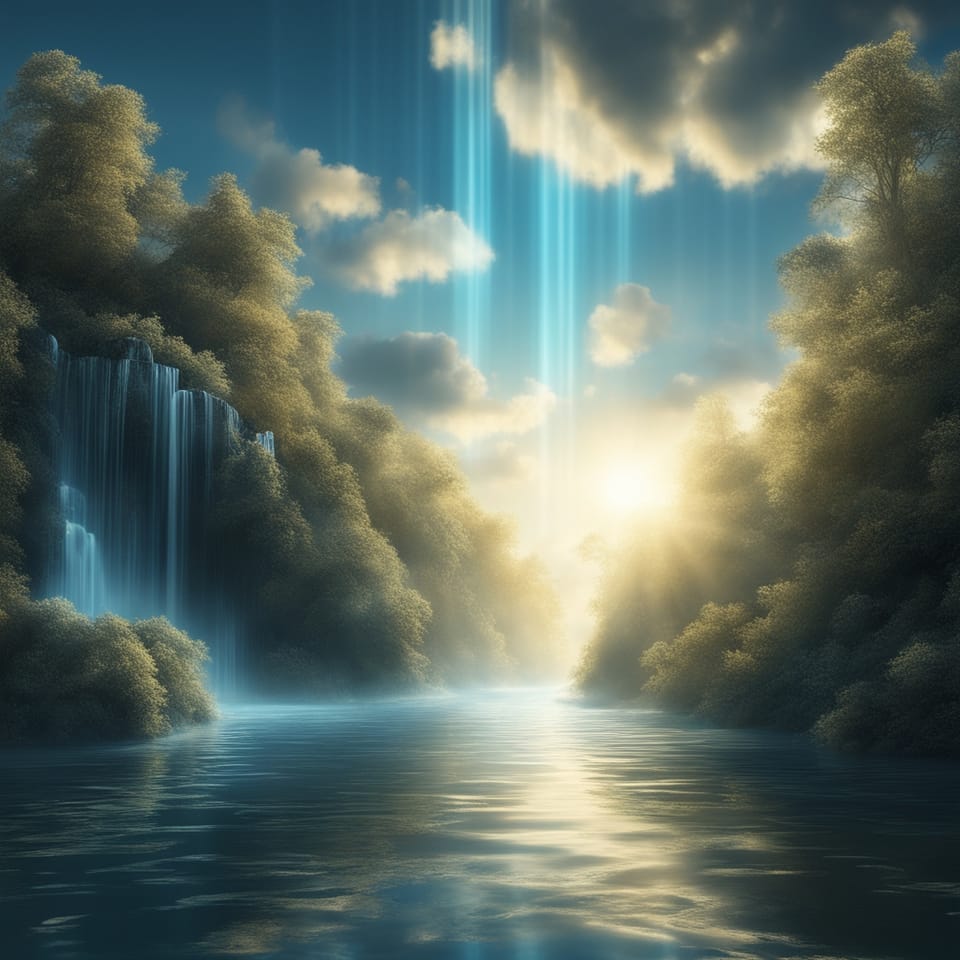
Java Source File Structure
Every Java source file will follow the same structure.
Package Declaration
Each file starts out with a package declaration. This is the package a class or interface is located in.
package press.bytesize.domain;
Import Statements
Import statements come after the package declaration. A Java source file will have zero or more import statements. Import statements allow you to reference a class by the class name instead of the fully qualified class name. An example of import statements in a Java source file is the following:
package press.bytesize.domain;
import java.util.List;
import java.time.LocalDateTime;
Declaration
A declaration in a Java source file can be a class, interface, annotation, enumeration, or record. For simplicity, only classes will be covered, but any declaration follows these same rules. Class declarations come after import statements. The class declaration starts with an access modifier. This can either be a public modifier or no modifier. No access modifier is package-private access. After the access modifier will be the class keyword, followed by the class name. After the class name is open and close braces. Inside of the braces is the class body, which contains various things such as variables, constructors, methods, etc.
package press.bytesize.domain;
import java.util.List;
import java.time.LocalDateTime;
public class User {
// ...
}
Source File Naming and Location
Java source files are named with the class, interface, annotation, enumeration, or record name followed by a .java extension. The location of this source file is associated with the package it is in.
package press.bytesize.domain;
public class User {
// ...
}
The source file above would be located in myproject/src/press/bytesize/domain/User.java where myproject is the root directory of your project.
Conclusion
The structure outlined in this article is the structure every Java file must follow. Every Java file must declare a class, interface, annotation, enumeration, or record.