Switch Statements in Swift
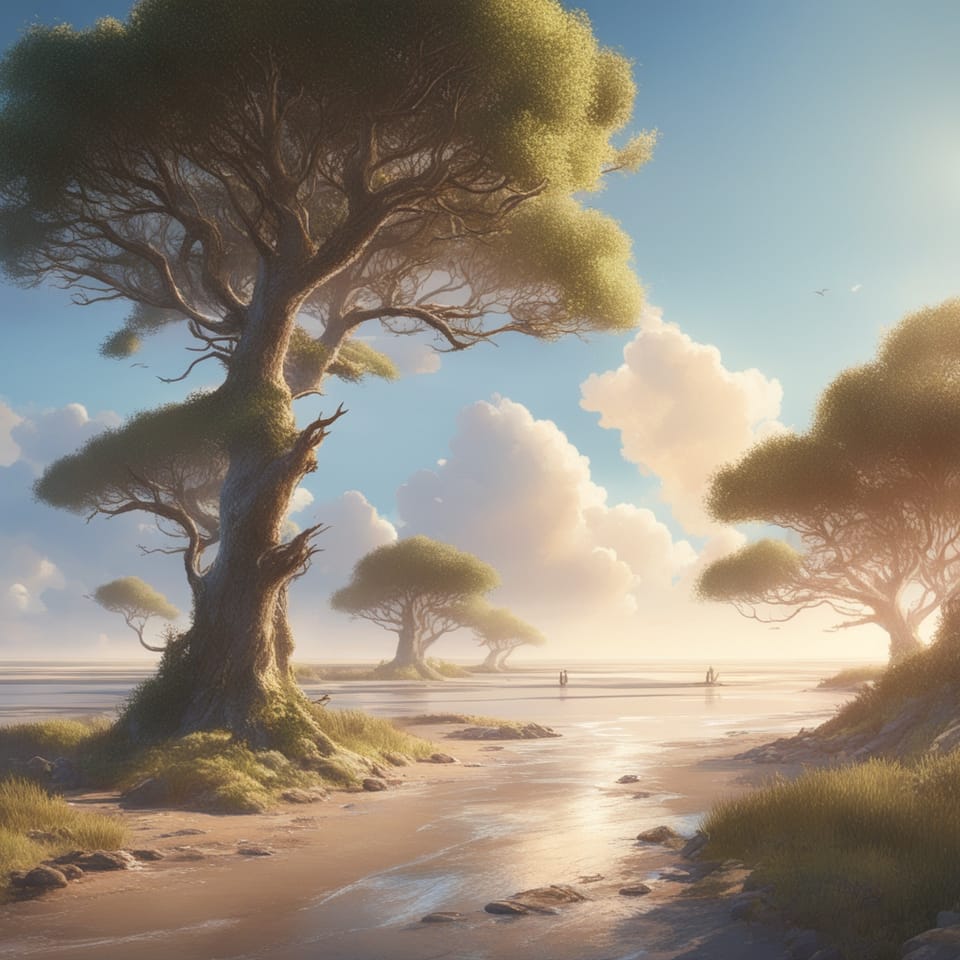
Switch Statements
Switch statements are similar to if statements, except instead of matching a condition, they match against one or more values. You can match both literal values and variables, and it can be used to match on any type. To keep things simple, this article is only going to show matching on numbers. Switch statements are written with the following:
let number = 10
switch number {
case 10:
print("Number is 10")
default:
print("Number is not 10")
}
A switch statement starts out with the switch keyword followed by the value you are going to match on. Each case to match is defined with the case keyword followed by the values you want to match. In this example, it matches a literal value of 10. The default clause is what will execute if nothing matches, similar to an else in an if/else. Switch statements must have a default clause, or it will result in a compiler error.
let number = 10
// Compiler error:
// switch must be exhaustive
// note: add a default clause
switch number {
case 10:
print("Number is 10")
}
Empty Default Clause
A default clause is required, but you may not need to put any code in it. Swift requires that there is always at least one statement. Because of this, the following code will generate a compiler error:
let number = 10
switch number {
case 10:
print("Number is 10")
// Compiler error:
// 'default' label in a 'switch' must have at least
// one executable statement
default:
}
When you don't need to do anything, you simply use the break keyword. This allows you to have an empty default clause and satisfy the compiler.
let number = 10
switch number {
case 10:
print("Number is 10")
default:
break
}
Falling Through
If you are coming from another language such as a C-like language, you will notice that switch statements do not have a break keyword to prevent fall through. This is because switch statements do not implicitly fall through. Instead, if you want it to fall through, you have to explicitly specify it. You do this with the fallthrough keyword.
switch number {
case 1:
fallthrough
case 2:
fallthrough
case 3:
print("Number is 1, 2, or 3")
case 4:
print("Number is 4")
default:
print("Unknown match")
}
If this matches 1, 2, or 3, it will print "Number is 1, 2, or 3" since it will fall through to case 3.
Matching Variables
So far, only literal values have been used, but you can also match using variables. Matching with a variable instead of a literal is written with the following:
let x = 1
let y = 2
let number = 2
switch number {
case x:
print("x matched")
case y:
print("y matched")
default:
break
}
Matching Multiple Values
Switch statements are restricted to just a single value. You can match one or more variables in each case. This is written with each value comma-separated.
let number = 5
switch number {
case 1, 2, 3:
print("1, 2, 3")
case 4, 5, 6:
print("4, 5, 6")
case 7, 8, 9:
print("7, 8, 9")
default
break
}
In this example, case 4, 5, 6 will match and print "4, 5, 6" to the console.
Matching Using Ranges
You can also use a range to match on a range of numbers. The previous example matched multiple numbers. Although that approach worked, the previous examples could be better written using a range.
let number = 5
switch number {
case 1...3:
print("1, 2, 3")
case 4...6:
print("4, 5, 6")
case 7...9:
print("7, 8, 9")
default
break
}
Switch Expressions
Switch statements are also switch expressions. This means that they return a value. Switch expressions are nice when used to assign a variable a value or return a value from a method. The following shows assigning a variable a value using a switch expression.
let number = 3
let numberAsString = switch number {
case 1:
"One"
case 2:
"Two"
case 3:
"Three"
default:
"Unknown"
}
When using a switch expression, you must return a value inside the default clause. If you don't, this will result in a compiler error:
let number = 3
let numberAsString = switch number {
case 1:
"One"
case 2:
"Two"
case 3:
"Three"
default:
// Compiler error:
// cannot 'break' in 'switch' when used as expression
break
}
Conclusion
Switch statements are a way to match one or more values and execute code based on a match. Unlike C-like languages, switch statements do not implicitly fall through. Switch statements can match one or more values of any type.