The Principle of One Operation Per Line of Code
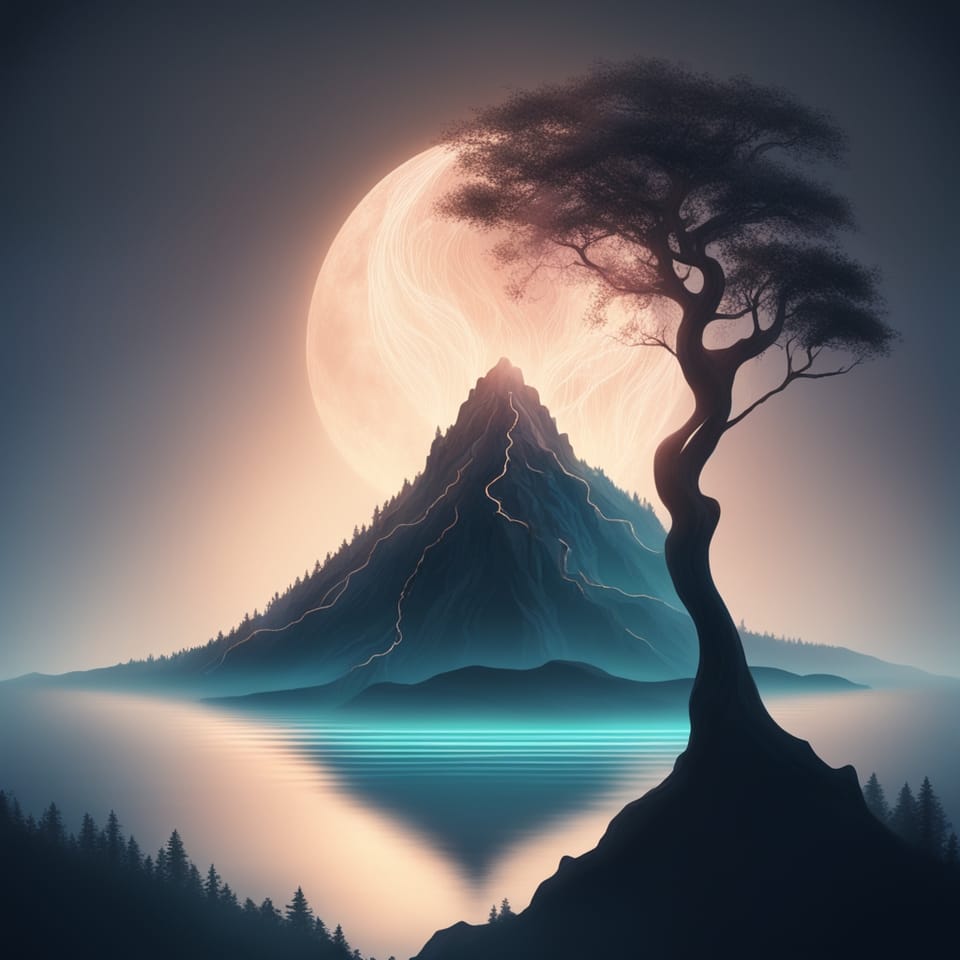
Doing One Thing
A method or function should do one thing. This is probably something you have heard, but what should a single line of code look like? A single line of code should perform one operation. It can feel great when you can do a lot in a single line of code. As tempting as this is, it is bad, and it should be avoided.
The programming language you use as a developer does not dictate the complexity as much as how it is used does. Using the Scala programming language as an example, it is easy to do a lot in one line of code, making it very complex. Because of the power behind the language, there are complaints about the complexity of the language. This doesn't make Scala a complex language as much as it makes it misused and abused. Misusing any tool will make the code harder to read, hard to modify, and hard to maintain. This can be done in any language.
One Operation Per Line of Code
A single line of code should be one operation. This can be an expression, a ternary, a method call, etc. One operation per line of code allows you to scan through the code to the relevant parts you are concerned with.
When invoking a method or function, this is probably when you see this principle violated the most. When passing arguments to a method or function, ternaries and expressions are probably the most misused. The following example intentionally has poorly named variables to show the complexity of doing more than one operation per line of code.
// Java code
doSomething((i + (x * 2)), a ? b : c);
This is complex, and this method only has two arguments passed to it. Even with better variable names, it would still be complex since there are three operations in this one line of code. This should be written as the following:
// Java code
final var y = i + (x * 2);
final var d = a ? b : c;
doSomething(y, d);
This now has one operation per line of code. Even with the poor variable names, this code is much simpler.
Duplication
Not doing one operation per line of code can influence code duplication or calling something more than once when it should only be called once.
// Java code
load(
isAuthenticated(user) ? user.theme() : Theme.Default,
isAuthenticated(user) ? user.config() : Config.Default
)
By doing one operation per line of code, the duplication can be eliminated with the following:
// Java code
final Theme theme;
final Config config;
if (isAuthenticated(user)) {
theme = user.theme();
config = user.config();
} else {
theme = Theme.Default;
config = Config.Default;
}
load(theme, config);
Each line of code in the previous example is doing one operation.
Chaining
When you have multiple calls chained together, such as what you would have with a builder pattern or working with collections, each call should be on its own line.
// Java code
final var loginForm = new FormBuilder()
.withTheme(Theme.Dark)
.withTitle("Login")
.withFields(emailAddress, password)
.build();
Never do this type of chaining with it formatted on the same line of code. This makes it difficult to read and modify. If you are chaining three times, it is probably better to have each on its own line of code. Chaining more than three times, always put them on new lines.
Debugging
Code that is doing too much in a single line becomes harder to debug. By keeping the code to one operation per line, you will make it easier to debug. You can set break points and easily see what variable values are. If a line of code is doing too many operations, you may have to break it up just to be able to debug it.
Conclusion
When you write a line of code, stick to a "less is more" approach with only having one operation per line of code. This increases readability and maintainability and will make the code easier to debug.