Things to Avoid When Writing if Statements
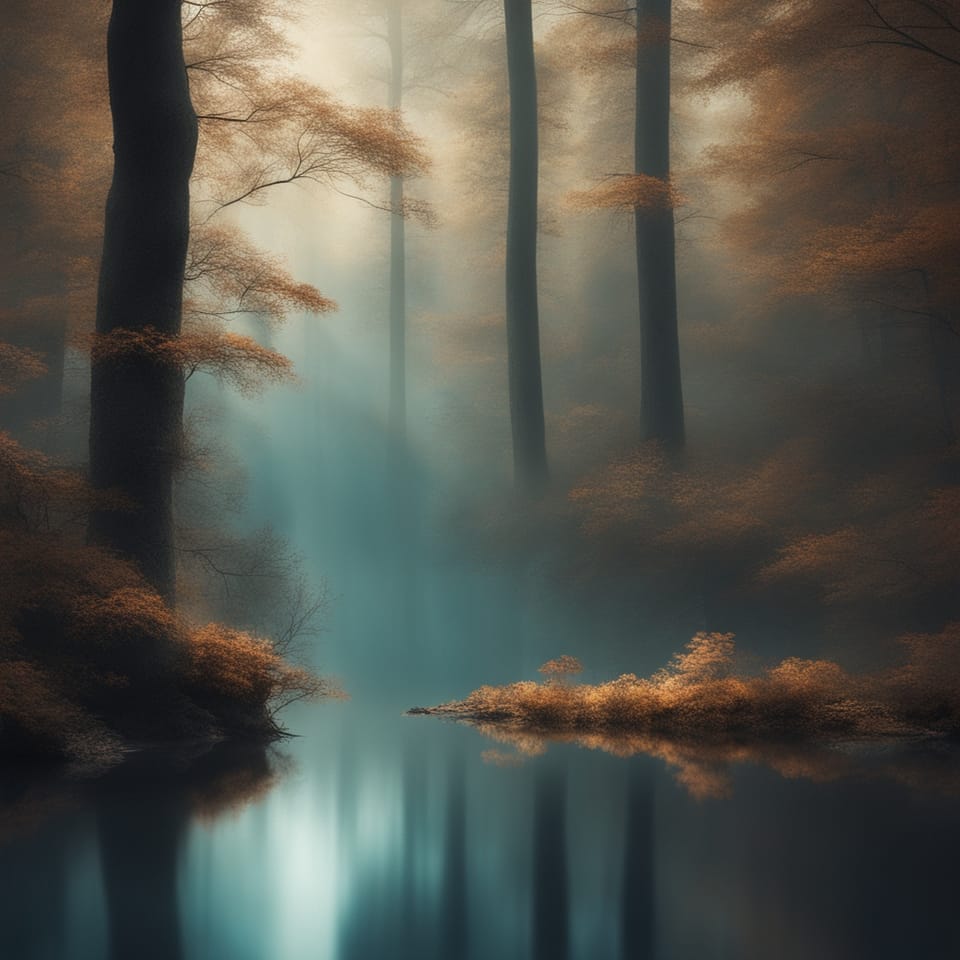
Boolean Constants
When writing if statements, never write them checking to see if they are equal to a boolean constant. For example:
// Java code
if (isHeaderRow == false) {
}
It's less readable and breaks the natural flow. In some languages, this can even be problematic. Always use just the conditional variable. If you need to reverse it, use ! instead. This is built into your language for a reason, and you should use it.
Nested if Statements
Nesting if statements are where you have an if statement within an if statement. For example:
// Java code
if (isCustomerFormValid) {
final Customer customer;
// This is a nested if statement
if (doesCustomerExist) {
throw new RuntimeException("Customer already exists");
} else {
customer = customerService.createCustomer(form);
}
//...
} else {
//...
}
There are multiple things wrong with nesting if statements. First, you now have more indentation in your code. More indentation means harder to read code. Indentation should be kept down to an absolute minimum. Indentation is not a problem with an if statement.
The second problem with nested if statements is the complexity it adds to the code. It will take longer to modify because it will take longer to understand. Third, a nested if statement is a concept that should be pulled out into a method. This will also document the code with code. The previous code would become:
// Java code
if (isCustomerFormValid) {
final Customer customer = createCustomer(form);
// ...
} else {
//...
}
private Customer createCustomer(final CustomerForm form) {
if (doesCustomerExist) {
throw new RuntimeException("Customer already exists");
} else {
return customerService.createCustomer(form);
}
}
Another example where you have a nested if statement is in the following code:
// Java code
if (usersFirstNameIsProvided) {
if (usersLastNameIsProvided) {
//...
}
}
The nested if statement in this example should be consolidated. This will still have the same short-circuit flow as the example above and will increase readability.
// Java code
final var isUsersFullNameProvided =
usersFirstNameIsProvided && usersLastNameIsProvided;
if (isUsersFullNameProvided) {
}
Ternary Operators
Ternary operators should be used in the case of a short one-line method or assigning a value to a variable. They're okay to use if they don't push your column character count beyond 80 characters. If they are larger than this, an if/else is going to be more readable. Don't ever try to break up a ternary operator over multiple lines. Keep it all on the same line. Also, just like if statements, never nest ternary operators! It's even worse than nested if statements.
Is it Okay to Omit Else With Code That Returns a Value or Throws an Exception?
Given the following code:
// Java code
if (a) {
return b;
}
return c;
It should really be written as an if/else like the following code:
// Java code
if (a) {
return b;
} else {
return c;
}
If statements show clearly the different paths the code can take. Looking at the previous code, you will know that there are two pathways without having to look at anything else. If you remove the else, it now causes two different types of thinking: the normal execution of the code and code taking different pathways depending on a conditional. This will take longer to understand, longer to modify, and increase the chances of introducing a bug.
This is not to be confused with code where all you need is an if, nor is it stating that an else is always required. This is code that has more than one pathway, yet the developer didn't write it that way.
Are Curly Braces Okay to Omit?
Although some languages allow you to omit the curly braces when the if statement is only one line, you should still write the curly braces. It is better to be consistent across the code. Also, if you have to modify that if statement later and make it more than one line, is the developer going to remember to add the braces when they are so easy to forget?
The only exception to this rule is if you are working with a language where you can write an if/else statement like a ternary operator. For example, in the Scala programming language, you can write the following:
// Scala code
if (x) y else z
If that is the case, go by the ternary operator rules.
When Should if Statements Not Be Used?
If statements should not be used when you are returning a boolean from a function or method or assigning a boolean value from another boolean value. Instead, you should use the result from the conditional test. Using the following examples:
// Java code
// Variable use case...
final boolean isUserLoggedIn;
if (user.isAuthenticated()) {
isUserLoggedIn = true;
} else {
isUserLoggedIn = false;
}
// Method use case...
public boolean isUserLoggedIn(final User user) {
if (user.isAuthenticated()) {
return true;
} else {
return false;
}
}
These are both bad ways of using if statements, and they are unnecessary. Both code examples above should be written like this:
// Java code
// Variable use case...
final var isUserLoggedIn = user.isAuthenticated();
// Method use case...
public boolean isUserLoggedIn(final User user) {
return user.isAuthenticated();
}
This is cleaner and much easier to read.
Conclusion
The rules for writing if statements are one of the simplest when it comes to programming constructs. Writing bad if statements will quickly take your application down the path of unreadable and difficult to manage code.