Tour of the JavaScript Programming Language
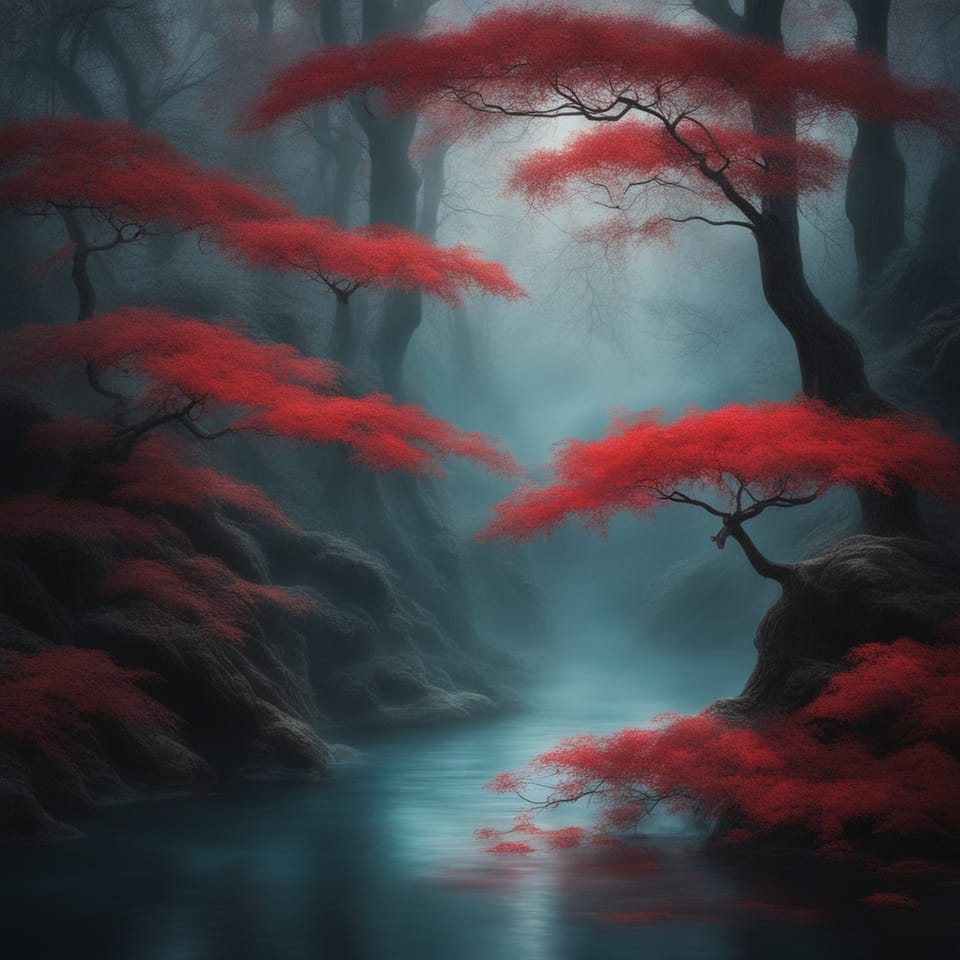
Why JavaScript
JavaScript is the language used for programming websites. JavaScript is also used in other areas, such as server-side programming and desktop programming.
JavaScript Naming
JavaScript is case-sensitive and generally uses camel case for names. Names can be a dollar sign ($), an underscore (_), or a-z, either in upper or lower case.
JavaScript Semicolons
Semicolons are optional unless you have multiple statements on the same line.
JavaScript Comments
Single line comments are written as follows and can also be added at the end of a line of code.
// This is a single line JavaScript comment.
Multi-line comments can be written as follows:
/*
This
is
a
multi-line
comment
*/
JavaScript Constants and Variables
Constants
Constants that cannot be reassigned are declared with const and follow the same scope rules as variables.
const i = 0;
// Not allowed!
i = 2;
Variables
Block-scoped variables that can be reassigned are declared with let.
let i = 0;
// Allowed
i = 1;
Global or function-scoped variables that can be reassigned are declared with var.
var i = 0;
// Allowed
i = 1;
You should only use const or let when declaring variables. Only use var if you have to write code for older technology that doesn't support let and const. Variables can also be declared without using a keyword if you are not using "use strict." This can result in unexpected behavior.
If a variable is declared but a value isn't assigned, it will have a type and a value of undefined.
// This is of type undefined and has a value of undefined
let name;
JavaScript Data Types
Booleans
Booleans are represented as either true or false.
const isEnabled = true
const isValid = false
Any variable of any data type that has a value is also true. Any variable of any data type that doesn't have a value is false.
Numbers
The number type in JavaScript represents both whole numbers and decimal numbers. Numbers in JavaScript are represented as a 64-bit double.
const num1 = 10
const num2 = 3.14
Invalid numbers are represented with the NaN (Not-a-Number) reserved word.
// Results in NaN
const number = 10 / "10"
You can check to see if a value is a valid number by using isNaN.
const isNumber = isNaN("10")
Variables can be converted to a number by using Number(). This will trim whitespace if the value has whitespace. If there are spaces in the value or the value passed in isn't a number, NaN will be returned.
const a = Number(true) // 1
const b = Number(" 87 ") // 87
const c = Number(" 87 87 ") // NaN
const d = Number("123.45") // 123.45
const e = Number("1,999") // NaN
Strings
Strings are assigned a value using quotes. Both single and double quotes can be used to assign a variable a string value.
const hello = 'hello'
const world = "world"
Strings can also be assigned values using backticks. This is used when you want to evaluate expressions or insert a variable value into the string.
const hello = 'Hello'
const world = 'World'
// Results in a value of 'Hello World'
const greeting = `${hello} ${world}`
const num1 = 4
const num2 = 3
// Value will be "Total is: 7"
const value = `Total is: ${num1 + num2}`
Special characters can be escaped by using \.
const reply = 'I can\'t make it tonight'
Variables can be converted to strings using String().
String(true) // Returns "true"
String(89) // Returns "89"
Arrays
Arrays are collections you use when you need to access values by index. Indexes start at zero.
Arrays defined as const cannot be reassigned. The values inside the array are not constant.
Arrays can be created with the following:
const config = [
true,
10,
'user-name'
]
You can create an array and add values to it later.
const config = []
config[0] = true
config[1] = 10
config[2] = 'user-name'
Values can be accessed and mutated with the index you are wanting to access or mutate.
const colors = [
'red',
'yellow',
'blue'
]
// Value will be 'yellow'
const selection = colors[1]
// index 0 is changed from 'red' to 'purple'
colors[0] = 'purple'
Values can be added to the end of an array with the push() function.
const colors = ['red']
colors.push('yellow')
colors.push('blue')
You can get the size of the array with the length property.
myArray.length
JavaScript Operators
JavaScript uses the same common operators as other programming languages, with a few additional operators. To keep this article short, only the differences will be covered.
Comparison Operators
JavaScript has both an == and an ===. Double equals checks to see if the value is equal. The triple equals check checks to see if the value and the data type are equal. Generally, you want to use triple equals.
5 == "5" // Returns true
5 == 5 // Returns true
10 != 10 // Returns false
5 === "5" // Returns false
5 === 5 // Returns true
10 !== 10 // Returns false
typeof
Typeof is an operator that returns a string of what the data type is.
typeof true // Returns "boolean"
typeof 8 // Returns "number"
typeof "name" // Returns "string"
typeof () => {} // Returns "function"
typeof [1, 2] // Returns "object"
typeof {name: "Bob"} // Returns "object"
instanceof
instanceof returns true if the date type is the specified data type.
const colors = ['Red', 'Yellow', 'Green']
// Returns true
if (colors instanceof Array) {
}
// Returns false
if (colors instanceof Number) {
}
JavaScript if Statements
If statements can be written with the following:
if (condition1) {
// ...
} else if (condition2) {
// ...
} else if (condition3 {
// ...
} else {
// ...
}
If statements can have zero or more else if blocks and zero or one else blocks.
The ternary conditional operator is supported in JavaScript and can be written with the following:
const min = a < b ? a : b
JavaScript Switch Statements
Switch statements are written with the following:
switch (value) {
case 'green':
// ...
break;
case 'blue':
// ...
break;
default:
// executes if nothing was matched
}
To stop the execution of code in a case block, use the break keyword. If the break keyword isn't used, the code will continue to execute each case, whether it matches or not. This is useful when you want to reuse code.
switch (value) {
case 'green':
case 'red':
case 'black':
// ... executes if value is 'green', 'red' or 'black'
break;
case 'blue':
// ...
break;
default:
// ...
}
JavaScript Loops
Break Statement
The break keyword is used to break out of a loop.
// inside of a loop somewhere
if (myCondition) {
break;
}
Continue Statement
The continue keyword is used to end the current iteration of the loop and go to the next iteration.
// inside of a loop somewhere
if (myCondition) {
continue;
}
For Loop
For loops are written in C/Java syntax style.
for (let i = 0; i < 10; i++) {
// ...
}
There are three statements in a for loop. The first is the initialization statement. This is an optional statement that is used to define zero or more variables, such as your loop counter variable. This statement is called once before the loop executes.
The second statement is an optional condition. This is the condition that determines if the block of code inside the loop executes or not. If you do not provide a condition, you will have to break out of the loop using the break keyword.
The third statement is an optional statement to update the value of your counter variable. This executes after the code inside your loop executes.
For-in Loop
For in loops, loop through each property of an object.
const person {
firstName: 'Tim',
lastName: 'Smith',
}
for (let key in person) {
person[key] = ''
}
This will loop through each property and set each property to ''.
For-of Loop
The for of loop is used for iterating through the values of an array, set, map, or string.
const colors = ['red', 'yellow', 'green']
for (color of colors) {
// ...
}
While Loop
A while loop is a loop with only a condition. It will execute until the condition is false.
while (condition) {
// ...
}
Do While Loop
A while loop and a do while loop are the same thing, with one exception. A do while loop will always execute an iteration once before evaluating the condition.
do {
// ...
} while (condition)
JavaScript Error Handling
Throw
Errors can be thrown using the throw keyword followed by a boolean, number, string, or object.
throw false;
throw 777;
throw "error";
throw {name: "name"}
try/catch/finally
When executing code that can throw errors, you can handle the errors using a try/catch/finally block. A try statement allows you to try to execute code that may throw an error. A catch statement is a block of code that executes if an error is thrown. A finally statement is a block of code that executes regardless of whether an error occurred or not and will execute after the catch if an error does occur.
try {
// code that might throw an error
} catch (err) {
// code that executes if an error is thrown
} finally {
// code that executes regardless of an error thrown
}
JavaScript Collections
Sets
Sets in JavaScript are collections of unique items sorted by the order in which each item was added. You can create a Set of items using the following:
const colors = new Set(['red', 'green'])
Items may be added after the set is created using the add method.
const colors = new Set()
colors.add('red')
colors.add('green')
Items can be removed using the delete method.
colors.delete('red')
You can loop through the values of a set using the forEach method.
colors.forEach(
color => // ... do something
)
You can check to see if a Set contains a value using the has method.
if (colors.has('green')) {
// ...
}
You can get the size of a Set with the size property.
const size = colors.size
Maps
Maps in JavaScript are a key/value collection sorted by the order in which each item was added. You can create a Map with items using the following:
const map = new Map([
['a', 'red'],
['b', 'green'],
['c', 'blue']
])
Maps can have values added after the map is created using the set method.
const map = new Map()
map.set('a', 'red')
map.set('b', 'green')
map.set('c', 'blue')
Items can be removed by passing the key to the delete method.
map.delete('b')
You can loop through the values of a map using the forEach method.
map.forEach(
(key, value) => // ... do something
)
You can check to see if a Map contains a value using the has method.
if (map.has('b')) {
// ...
}
You can get the size of a Map with the size property.
const size = map.size
JavaScript Functions
A function can be defined with zero or more parameters. Function names follow the same rules as variable names.
function hello() {
}
function add(num1, num2) {
}
Functions can be defined inside of functions.
function a() {
function b() {
function c() {
function d() {
}
}
function e() {
}
}
}
Functions can return values using the return statement.
function add(num1, num2) {
return num1 + num2
}
Functions can have any number of return statements. Functions without return statements will return the value undefined.
Functions can be passed around as variables or invoked with the () operator.
// Declare a function
function hello() {
return "Hello World!"
}
// declare a variable and assign it the value of hello
const s = hello
// invoke. Both of these call the same function
const greeting1 = s()
const greeting2 = hello()
Functions can be defined using an arrow function as well.
const addNumbers = (num1, num2) => num1 + num2;
JavaScript Objects
Objects in JavaScript are key value pairs. Values can be anything a normal variable can be. An object's properties can be accessed inside the object using the this keyword.
const customer = {
id: 99,
firstName: 'Mike',
lastName: 'Smith',
active: true,
fullName(): function() {
return this.firstName + ' ' + this.lastName
}
}
Properties can be accessed in two different ways.
// By property
person.firstName
// or by property name
person['firstName']
JavaScript Object Notation (JSON)
JSON is commonly used for transferring data from a server to a client. JSON can easily be converted into a JavaScript object using the following:
const user = JSON.parse(jsonText);
For more on the JSON standard, click here.
JavaScript Classes
Classes are templates for creating instances of objects. Instances are created using the new keyword.
Constructors
Constructors are the code that is called when you create a new object using the new keyword. Constructors are optional and are only needed when you need to execute code when the instance is created.
class User {
constructor() {
// ...
}
}
const user = new User();
Constructors can have parameters passed into them.
class User {
constructor(firstName, lastName) {
// ...
}
}
Methods
Methods are defined similarly to functions, just without using the function keyword. Methods can have parameters just like functions.
class User {
hello() {
return "Hello World!";
}
}
const user = new User();
user.hello();
Properties
Classes can have properties defined.
class User {
name;
constructor(name) {
this.name = name;
}
hello() {
return "Hello " + this.name;
}
}
const user = new User("Bob");
user.name;
Properties can have getters and setters as well.
class User {
name;
get fullName() {
return this.name;
}
set fullName(value) {
this.name = value
}
}
Static Methods
Classes can have static methods that are called on the class and not an instance of a class.
class User {
static hello() {
return "Hello World";
}
}
User.hello();
Encapsulation
Methods and variables can be private in JavaScript by prefixing them with #.
class User {
// private
#firstName;
// private
#lastName;
constructor(firstName, lastName) {
this.#firstName = firstName;
this.#lastName = lastName;
}
// private
#buildName() {
return this.#firstName + " " + this.#lastName;
}
// public
name() {
return this.#buildName();
}
}
Inheritance
Classes can inherit from other classes using the extends keyword. The constructor of the class it is inheriting from can be called with the super() function inside the constructor. The call to super() must be the first call in the superclass constructor if you are going to use it.
class User {
name;
constructor(name) {
this.name = name;
}
}
class Admin extends User {
constructor(name) {
// Calls Users constructor
super(name);
}
}
Conclusion
JavaScript is a powerful language with some unique features. This only covers the important common features. JavaScript does have more that you can do with it and can change depending on the engine that is executing it.