Tuples in Swift
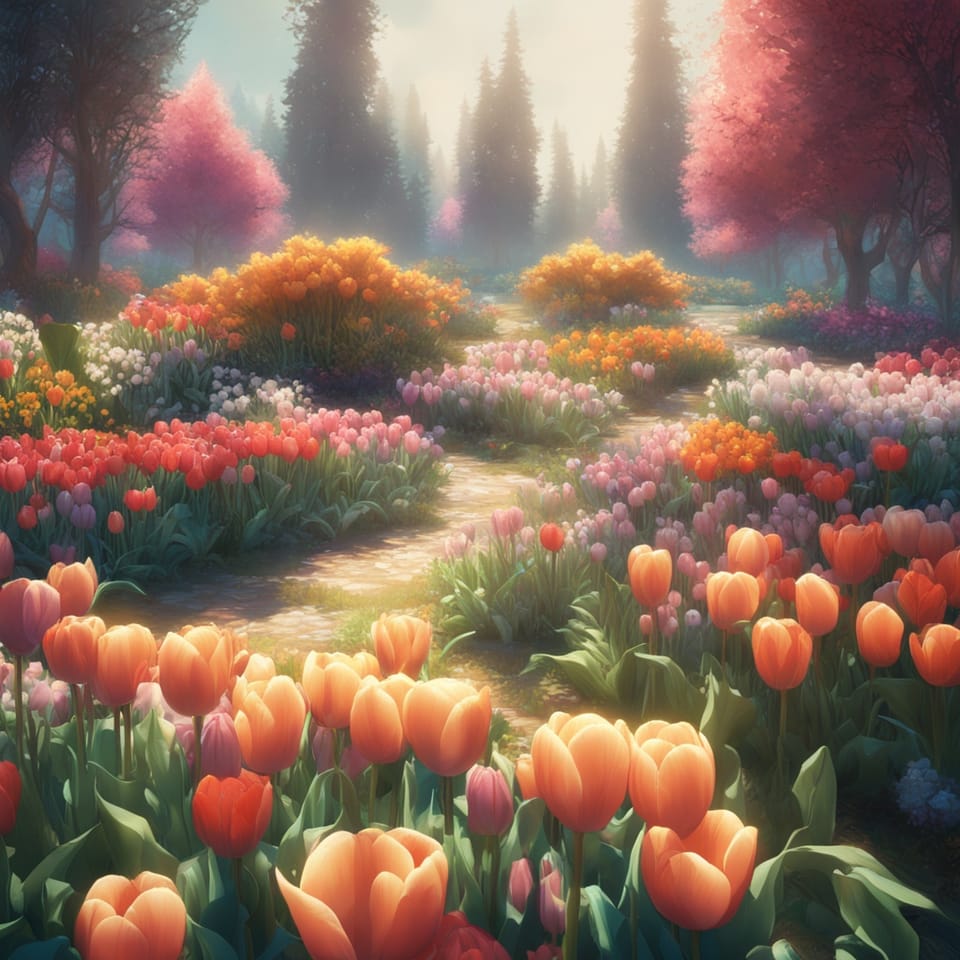
What Are Tuples?
Tuples are a type that allow you to define multiple values. Tuple values can be the same type or different types. They are either mutable or immutable depending on if they are defined using the let or var keywords.
Using Tuples
Tuples can use type inference or provide the type annotation for each type. Tuples are defined with the following:
// Type inference
let point = (5, 9)
// Type annotation
let point: (Int, Int) = (5, 9)
Tuple values can be a literal value or use a variable for the value.
let x = 5
let y = 9
let point = (x, y)
Accessing a tuples values is done with the following:
let point = (5, 9)
print(point.0) // 5
print(point.1) // 9
If a tuple is defined with the let keyword, values cannot be reassigned. If they are defined with the var keyword, the values can be reassigned.
var point = (0, 0)
point.0 = 5
The values in a tuple can be named, making the code much more readable. This allows you to access each value by the name instead of the index.
let point = (x: 5, y: 9)
print(point.x)
print(point.y)
You can also provide the type annotation when naming tuple values.
let point: (x: Int, y: Int) = (5, 9)
Tuples don't have to be the same type, and each value can be a different type.
let point = (x: 0, y: 0, description: "Starting position")
This tuple is two Ints and a String.
Using Tuples With Functions
Tuples can be passed as arguments into a function or returned from a function. Depending on how you define the tuple in the function signature, will determine how it can be used inside the function or when it is returned from a function.
Passing in a tuple to a function can be done with the following:
func tupleFunc(_ tuple: (Int, Int)) {
print(tuple.0)
print(tuple.1)
}
If you provide a name for each of the items in the tuple, you can reference those values by name inside the function.
func tupleFunc(_ tuple: (x: Int, y: Int)) {
print(tuple.x)
print(tuple.y)
}
Returning a tuple from a function can be done with the following:
func returnTuple() -> (Int, Int) {
return (0, 0)
}
let tuple = returnTuple()
print(tuple.0)
print(tuple.1)
Just like with function parameters, you can provide a name to each of the values so they can be referenced by name.
func returnTuple() -> (x: Int, y: Int) {
return (0, 0)
}
let tuple = returnTuple()
print(tuple.x)
print(tuple.y)
Conclusion
Tuples allow you to store a variable with multiple values. Each value can be a different data type, and they can be named to make the code more readable. Tuples will be either mutable or immutable depending on if they are declared using the let keyword or the var keyword.