Type Conversion in Java
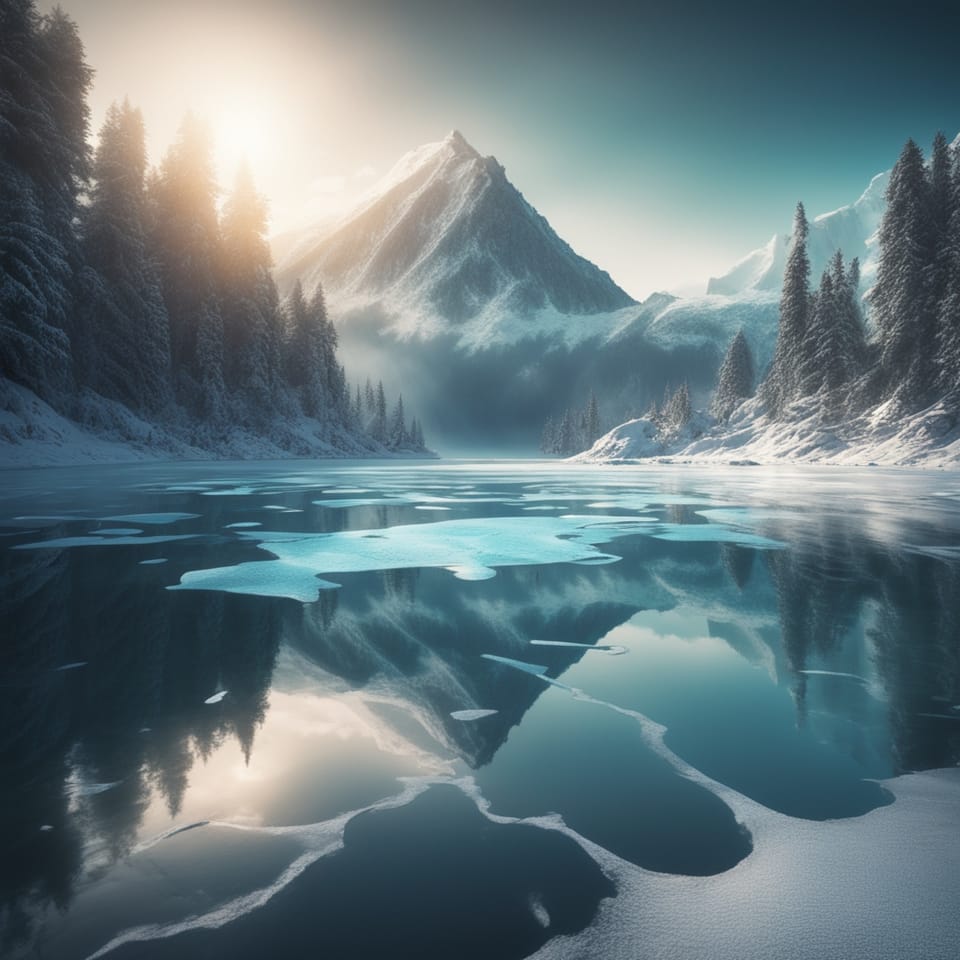
Widening Type Conversion (Automatic Conversion)
Widening type conversion happens automatically when you convert a smaller primitive number into a large primitive number. The order is byte -> short -> char -> int -> long -> float -> double.
final short x = 10;
final int y = x;
final long z = y;
No conversion by the developer is needed for this.
Narrowing Type Conversion (Explicit Conversion)
Narrowing type conversion is explicitly done by the developer and is needed when going from a bigger to a smaller primitive. The order is the same but reversed: double -> float -> long -> int -> char -> short -> byte. To cast from a bigger to a smaller primitive, you would write the following:
final double x = 10;
final int y = (int) x;
final byte z = (byte) x;
When going from a bigger type to a smaller type, there can be loss of data if the value is too big for the type you are converting to.
Converting to a String
You can convert from one type to a String using the String.valueOf() method.
final String ten = String.valueOf(10); // int
final String object = String.valueOf(object); // Object
final String isValid = String.valueOf(true); // boolean
If you pass an Object to this method, you will either get the value "null" if the object is null or it will call the toString() method on the object.
String to Character
If a String is a single character, you can convert it using the charAt() method, grabbing the first character.
final char c = "c".charAt(0);
If a String is multiple characters, you can convert it into a char array using the toCharArray() method.
final char[] c = "abc".toCharArray();
String to Boolean
Strings can be converted to booleans in two different ways, depending on if you want the wrapper class or the primitive type.
final boolean a = Boolean.parseBoolean("true");
final Boolean b = Boolean.valueOf("true");
Both methods ignore whether the value is uppercase or lowercase. If the value passed in equals "true" the method will return true. Otherwise they will return false, including a null value.
String to Number
Strings can be converted to the number primitive or wrapper class, similar to how you convert strings to booleans. Both of these methods can throw a NumberFormatException if the String passed in isn't a number. To convert a String to a primitive number type, you can use the following:
final byte b = Byte.parseByte("10");
final short s = Short.parseShort("10");
final int i = Integer.parseInt("10");
final long l = Long.parseLong("10");
final float f = Float.parseFloat("10");
final double d = Double.parseDouble("10");
To convert a String to a wrapper class, use the valueOf() method on the class you want the String converted to.
final Byte b = Byte.valueOf("10");
final Short s = Short.valueOf("10");
final Integer i = Integer.valueOf("10");
final Long l = Long.valueOf("10");
final Float f = Float.valueOf("10");
final Double d = Double.valueOf("10");
Conclusion
Converting between two different types is done in one of two ways. Either using an explicit cast such as (long) or going through a method. Converting from an object to another object or an object to a primitive is done through a method.