Type Conversion in Swift
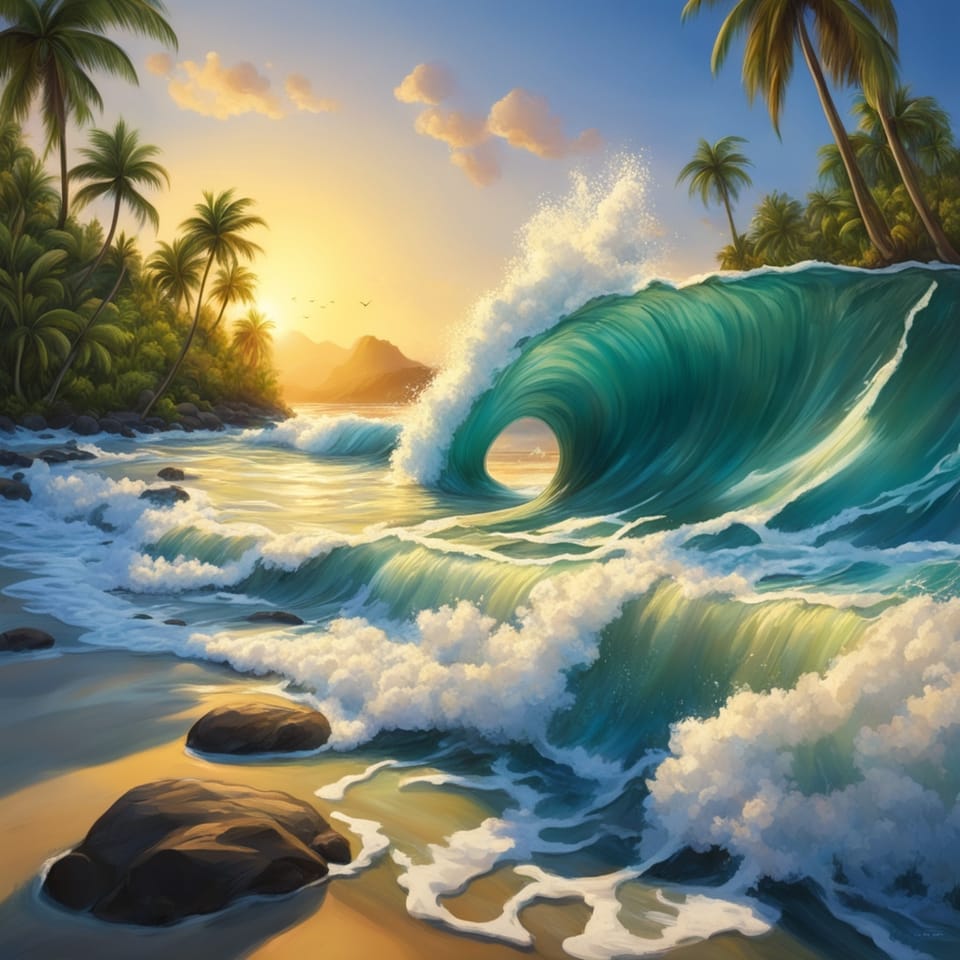
Data Types
It is common to have to convert from one data type, such as an Int to a String. Swift is strict with data types. You cannot perform the following without a compiler error.
let x: Double = 5
let y: Int = 2
// Compiler Error:
// binary operator '+' cannot be applied to operands of
// type 'Double' and 'Int'
print(x + y)
This same problem can be seen in the following example:
let text: String = "My age is "
let age: Int = 30
// Compiler Error:
// binary operator '+' cannot be applied to operands of
// type 'String' and 'Int
print(text + age)
Swift requires you to be explicit with the data types. In the first example, would you want a result of an Int or a Double? In the second example, it seems more reasonable because you are concatenating a String but this isn't allowed in Swift. You can only perform operations on the same type. This is where type conversion comes into play.
Type conversations are done with the following: Bool(), Character(), Double(), Float(), Int(), and String().
Converting Number Data Types
The first example in this article shows how you cannot perform operations on two different numeric types. To get around this, you must convert to the same type depending on what data type you are working with. This is done using Int(), Float(), or Double() depending on what data type you want.
let x: Double = 5
let y: Double 6
let z: Int = Int(x) + Int(y)
This same thing can be done with any of the numeric types,
Converting a Type to String
Converting different data types to a String can easily be done.
let booleanValue: Boolean = true
let characterValue: Character = "y"
let intValue: Int = 10
let doubleValue: Double = 1.02
let floatValue: Float = 1.11
let booleanAsString = String(booleanValue)
let characterAsString = String(characterValue)
let intAsString = String(intValue)
let doubleAsString = String(doubleValue)
let floatAsValue = String(floatValue)
Converting a String to Another Type
When converting a String to another type, most of the time it will return an Option of that type.
Convert to Bool
When converting a String to a Bool, it will return an Option of the converted value.
let asString: String = "true"
let trueBool = Bool(asString) // Option(true)
let falseBool = Bool("false") // Option(false)
let nonBool = Bool("t") // nil
Convert to Character
When converting a String to a Character, if there is more than one character, it will result in a fatal error. If it is a single character, it will be converted to a String.
let asString: String = "x"
let asCharacter = Character(characterValue)
Convert to Float or Double
When converting a String to a Float or Double, it will return an Option. If it isn't a number, it will return nil.
let aDouble: String = "1.023"
let aNonDouble: String = "string"
Double(aDouble) // Option(1.023)
Double(aNonDouble) // nil
If it is a decimal and it is bigger than what a Float or Double allows, it will be rounded.
let asString: String = "1.123456789"
let asDouble = Double(asString) // Option(1.123456789)
let asFloat = Float(asString) // Option(1.1234568)
Convert to Int
When converting a String to an Int, it will return an Option.
let doubleAsString: String = "1.01"
let intAsString: String = "47"
let nonIntAsString: String = "Hello World"
Int(doubleAsString) // nil
Int(intAsString) // Option(47)
Int(nonIntAsString) // nil
Conclusion
Swift enforces you to do operations only on the same type. Because of this, you must ensure that the type you are applying an operation to is the same type. To do this you use type conversion.