Variables in Java
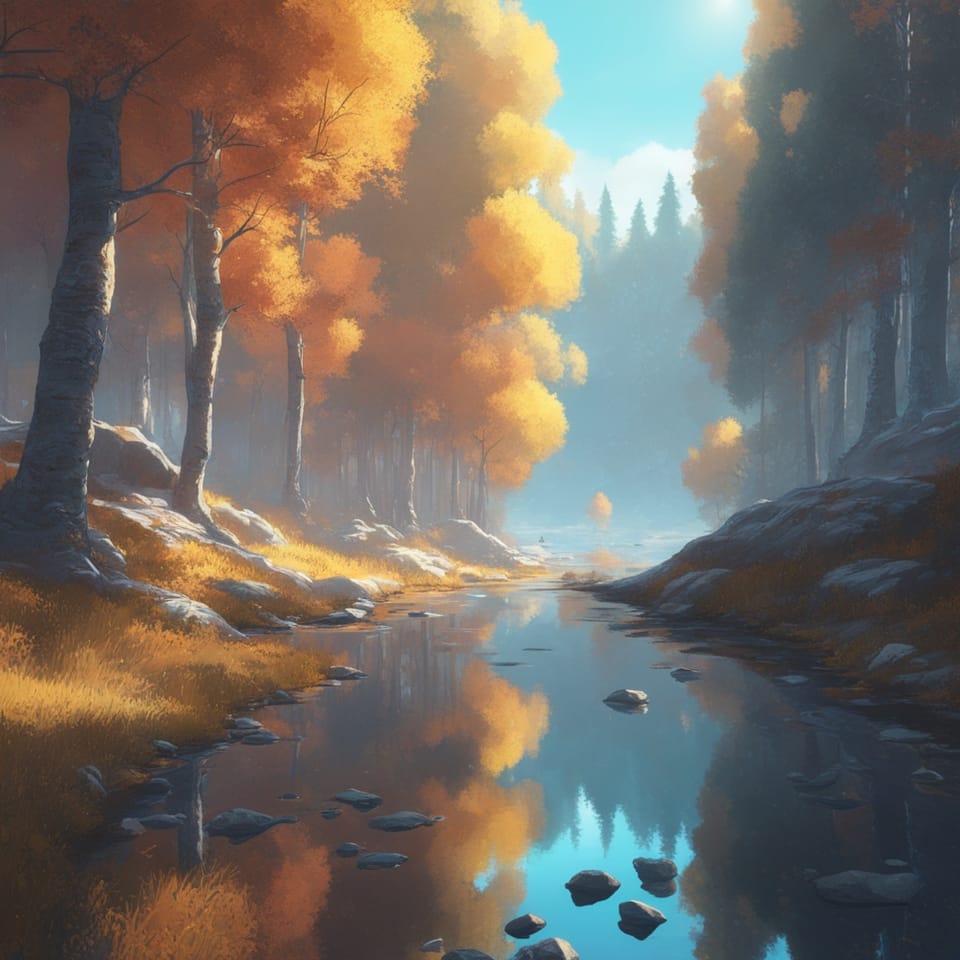
Variable Types
Java supports four different types of variables.
- Local Variables: These are variables defined inside of a constructor or method. They can be mutable or immutable.
- Instance Variables: Variables defined at the class level. They can be mutable or immutable.
- Class Variables: Variables defined at the class level marked as static and only have one copy.
- Constants: Variables defined at the class level marked as static final and only have one copy.
Variables
Each variable type defined above is declared the same way. Each variable starts with a type, followed by a name and an optional value assigned to it. The type can be a primitive value such as a char, byte, short, int, long, boolean, float, double, or a class type.
String greeting = "Hello World";
Variables are mutable and can be reassigned a value. Variable names are written using camel case. Variable names start with a letter or an underscore. After this they can contain letters or numbers but have to start with a letter or underscore. Variable names cannot be named using any Java keywords.
Variable Names
Variable names are named using camel case convention. Names must start with either a letter or an underscore. Names can contain letters, numbers, dollar signs, and underscores.
String fullDescription = "description";
Immutable Variables
Immutable variables are variables that cannot be reassigned a value once the value is assigned. Immutable variables are declared the same as mutable variables, except they start with final.
final String greeting = "Hello World";
Type Inference
Type inference was introduced into Java 10. This feature is only allowed for local variables. Type inference should only be used when the variable type is obvious. Using type inference, you can declare a variable omitting the type and replacing it with the var keyword.
var greeting = "Hello World";
Variables using type inference can also be marked as final.
final var greeting = "Hello World";
Conclusion
This covers the basics of variables in Java. Variables start with an optional final keyword depending on if they are mutable or immutable. After that follows the type and the variable name. You can then assign a value to the variable.