Variables in Swift
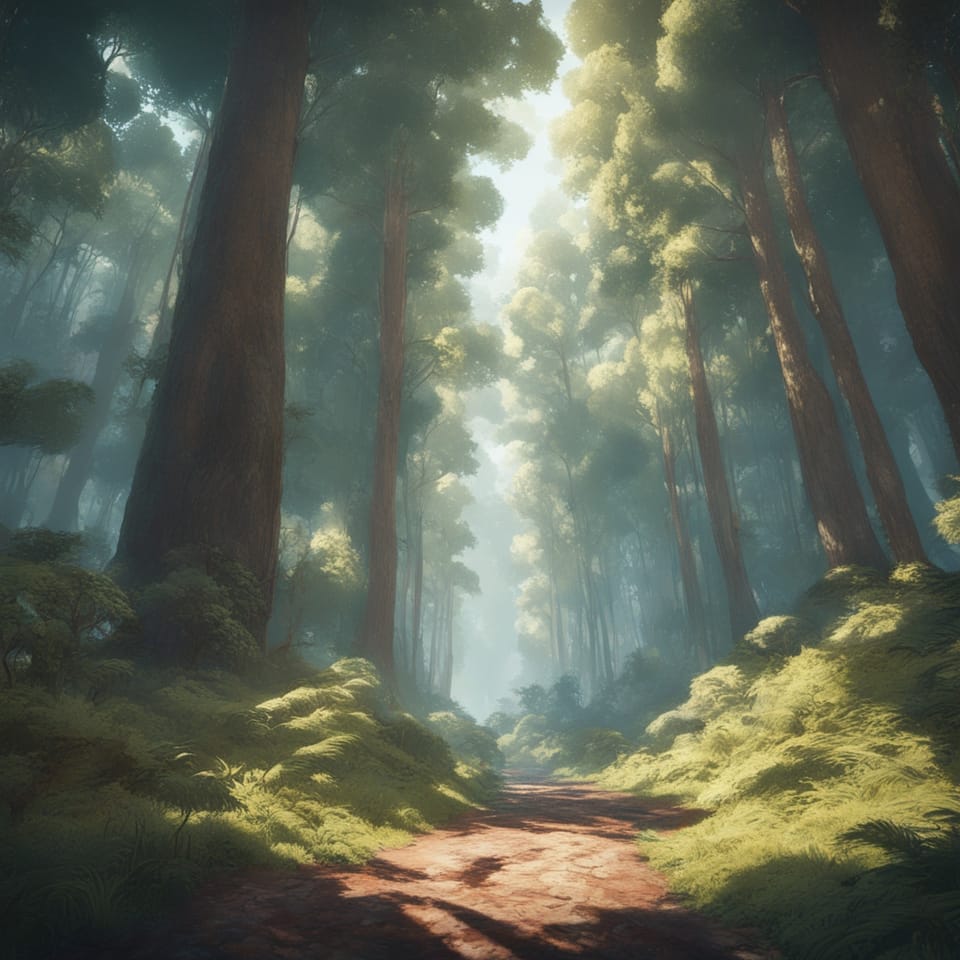
Variables
Variables are a way to name a piece of mutable data. Variables can be assigned a value when they are declared or assigned later on before they are used. Variables can be declared and assigned a value with the following:
var x = 10
Variables start with the var keyword, followed by a name and then assigned a value.
Swift uses type inference. Type inference is where it will infer the type so you do not have to provide the type. In the previous example, Swift will infer the type as an Int. You can provide the type annotation when declaring a variable.
var x: Int = 10
This is useful for both readability when it isn't obvious and when you want a different type than what Swift will infer. With the first example, the type annotation isn't needed because it is obvious that it is an Int. In other cases, it may not be obvious, and you will have to provide the type.
Variables can be declared and then later assigned. If you do this, you do have to provide a type annotation. Since there isn't a value assigned when the variable is defined, the type cannot be inferred, so it has to be provided.
var greeting: String
greeting = "Hello World"
Variables do not have a default value. Swift is strict about variable values. If you do not provide a value for a variable before you try to use it, it will result in a compiler error.
var x: Int
// Compiler Error:
// variable 'x' used before being initialized
print(x)
Variable Names
Variable names must start with a letter or underscore and are written using the camel case convention. Special characters such as !, #, @, etc. cannot be used in variable names.
let helloWorldGreeting = "Hello World"
let _name = "name"
Variable names also support Unicode characters.
let 😃 = "Smile"
Although this can be done, it should be avoided. It will make the code hard to write and hard to read. This makes more sense as a variable value and not a variable name.
Constants
Constants are immutable variables that cannot be reassigned a value after it is assigned a value. Constants are defined just like variables, except they use the let keyword instead of the var keyword.
let x = 10
Constants can be defined and then later assigned a value just like variables.
let x: Int
x = 10
Once a constant is assigned a value, it cannot be reassigned a value, or you will get a compiler error.
let x = 10
// Compiler Error:
// immutable value 'x' may only be initialized once
// note: change 'let' to 'var' to make it mutable
x = 11
Always try to use constants before using variables. If a variables value doesn't need to change, make it a constant.
Conclusion
Variables are used to store data. They can be constant or be allowed to be reassigned later. Use constants as much as you can and use variables only when the variable is going to be reassigned.