What Type of Comment Should You Use, and How Do You Format Them
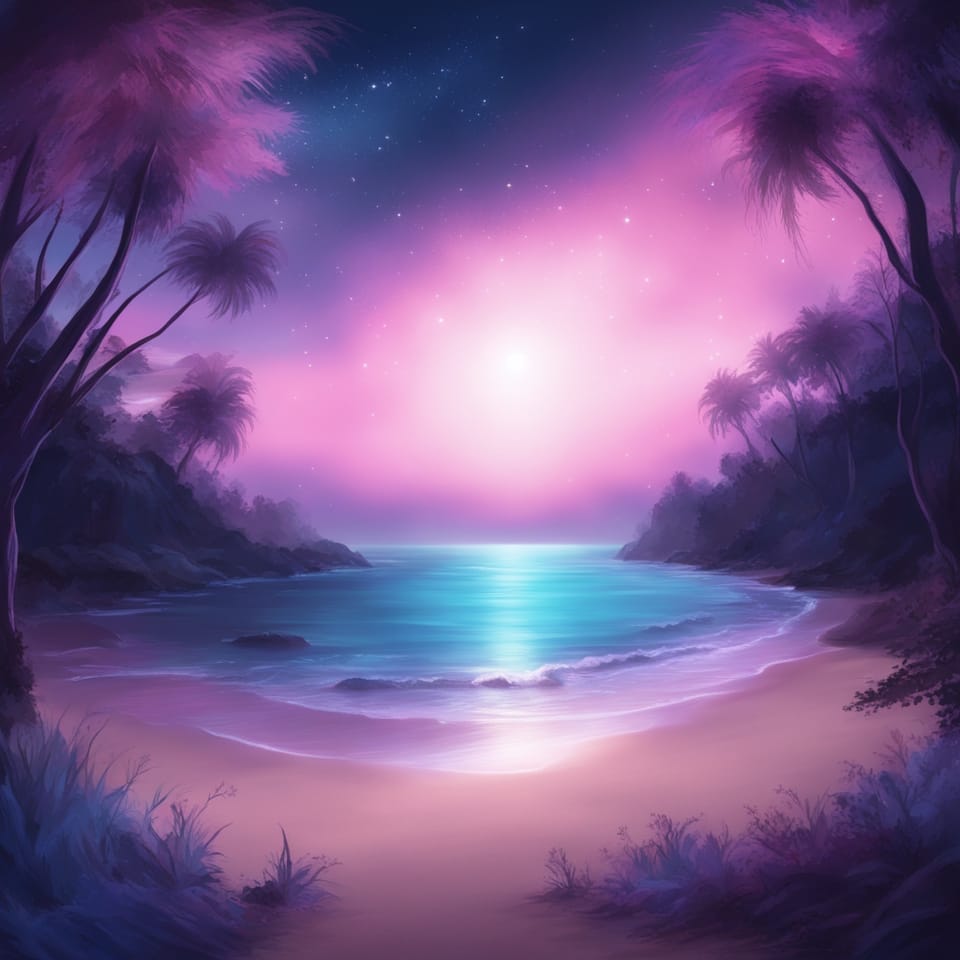
Different Comment Types
Comments in most languages usually come in three forms: single-line comments, multi-line comments, and documentation comments.
Starting a Comment
When writing a comment, don't write the comment character(s) and immediately start the comment. Instead, start the comment with a space. This will make the comment more readable.
//Don't do this
// Do this instead
Single-line Comments
Single-line comments should generally be written on their own line.
// Commenting something
final var id = 1;
If there is a line of code above a comment, consider adding a new line so the code and comment read better.
final var id = 1;
final var name = "Matt";
// Commenting something about the thumbnail image
final var thumbnail = Thumbnail.DEFAULT;
This code should be written as follows:
final var id = 1;
final var name = "Matt";
// Commenting something about the thumbnail image
final var thumbnail = Thumbnail.DEFAULT;
If the order doesn't matter, this code would be even simpler, written as follows:
// Commenting something about the thumbnail image
final var thumbnail = Thumbnail.DEFAULT;
final var id = 1;
final var name = "Matt";
Trailing Comments
Trailing comments are comments that come at the end of the line.
final var thumbnail = Thumbnail.DEFAULT; // trailing comment
In general, avoid using trailing comments. They can make lines too long and are harder to read. There are some cases where using a trailing comment would make the code and comment more readable, but use them sparingly and keep them short.
Multi-line Comments
Multi-line comments are comments that span over multiple lines. In the Java programming language, you can write a multi-line comment with the following:
/*
This is
a multi-line
comment in Java
*/
Multi-line comments aren't as common to see, even if the comment spans over multiple lines. Most teams would write the comment above with the following:
// This is
// a multi-line
// comment in Java
Multi-line comments are good to use when you need to have information stand out for whatever reason. One example of this would be legal information. Another use is if you have to document two separate things about the code.
/*
Due to legacy code support, we have to use a
legacy type for backwards compatibility
*/
/*
Version 10 introduced a bug so if the user is
coming from a previous version, we have to have
special handling here to account for the length
*/
final var somethingLegacy = "";
This helps separate the two topics, and by doing this with a single-line comment, they blend together more.
As a general rule, stick to using single-line comments even when the comment spans over multiple lines.
Documentation Comments
Documentation comments are comments used to document the public API (Application Programming Interface) and should be the only type of comment used for that type of documentation. In the Java programming language, these types of comments are referred to as Javadocs. An example of a documentation comment (Javadoc) in Java would be.
/**
* Description of the method/function.
* @param num1 Description of num1 parameter.
* @param num2 Description of num2 parameter.
* @return Description of what it returns.
*/
public int add(final int num1, final int num2) {
return num1 + num2;
}
Make sure you stick to your programming language's standard for how these comments should be written and formatted.
Punctuation in Comments
Documentation comments should always have good punctuation. Single line comments should start with a capital letter but can be more loose on the standards, such as not putting a period at the end of the comment. It is going to be less common for a comment to come in the form where they'd require a question mark or exclamation mark. Punctuation marks like commas should still be used since this increases readability.
Commenting Lines of Code
When you need to comment out a line of code or multiple lines of code, use a single-line comment. Generally, your IDE is built to use single-line comments when you use the IDE to comment out a line of code. Multiple lines of code can usually be highlighted, and then your IDE can comment them out. If you use a multi-line comment for this, it can make uncommenting the code more difficult, especially if the code that needs to be uncommented is in the middle.
Conclusion
Whatever you and your team decide for comments, stay consistent. In general, though, stick to your language standards and use single comments and documentation comments.