While Loops in Swift
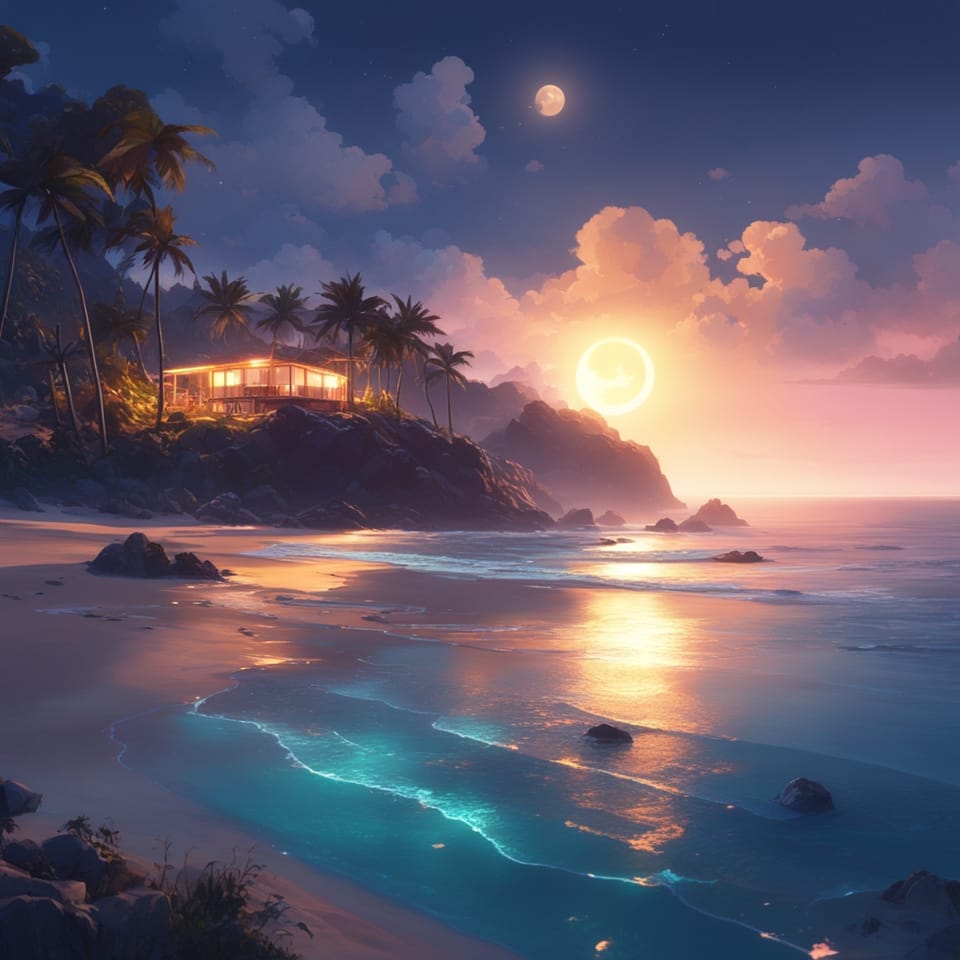
What Are While Loops?
A while loop is a way to execute a block of code until a condition is met. It first checks to see if a condition is true. If that condition evaluates to true, it will execute an iteration of that loop. Once the condition evaluates to false, it will no longer execute any more iterations of the loop.
While Loop Syntax
While loops are written with the following syntax:
while i != 5 {
print(i)
}
A while loop starts with the while keyword. After the while keyword follows the condition that must evaluate to true to execute an additional iteration of the loop. The block of code to execute is defined inside of braces.
With the loop in the previous example, since it is using a counter, you will need to update the counter in the loop, or it will result in an infinite loop.
var counter = 0
while counter != 5 {
print(counter)
counter += 1
}
This while loop will print out the following:
0
1
2
3
4
While loops are best used when working with an unknown number of iterations. The following example shows a while loop used this way.
while mugContainsCoffee() {
sipCoffee()
}
Conclusion
While loops execute a block of code while a condition is true. Once that condition no longer evaluates to true, it will stop executing the while loop.