Why Are Loops Needed in Programming
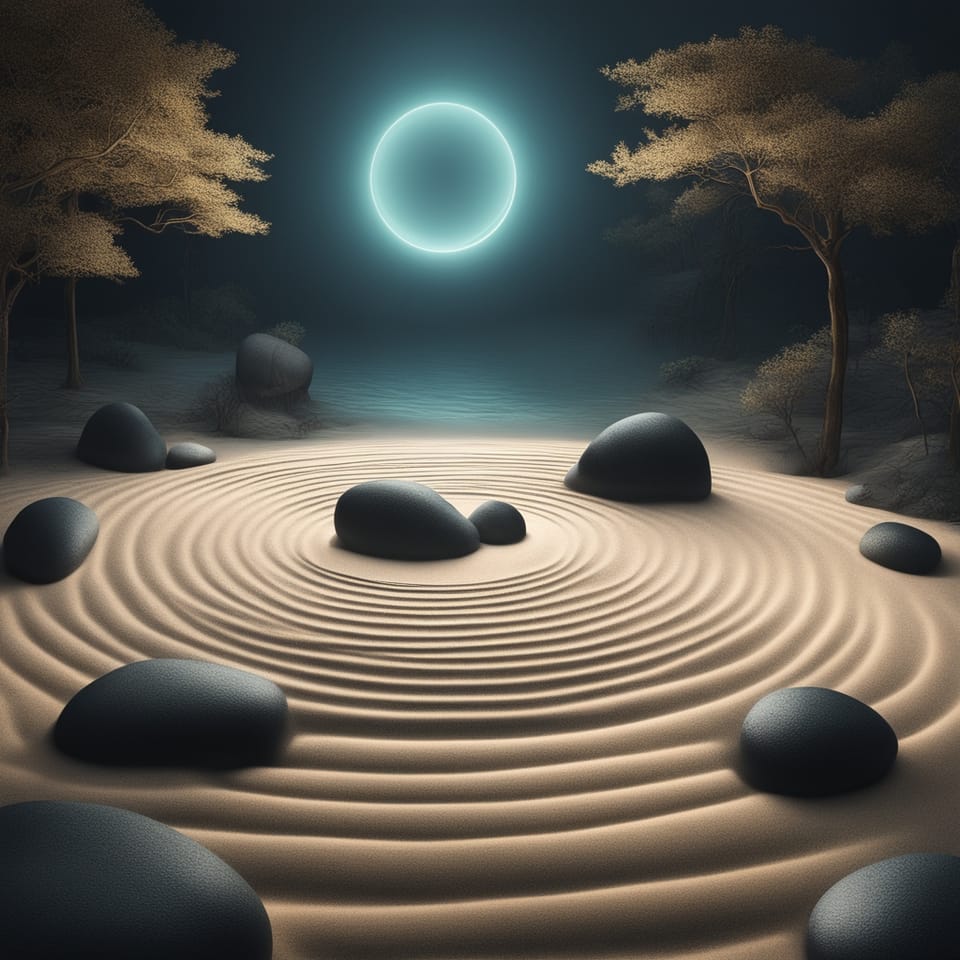
Repeating a Certain Number of Times
In programming, it is common to have to repeatedly execute a block of code. This can be accomplished by repeating the block of code over and over. For example, if you had a method you needed to call three times, you could do it with the following:
doSomething();
doSomething();
doSomething();
However, this is not a good way of doing things. It's static. What if you had a dynamic number of times you needed to perform an operation? You couldn't do what was shown in the previous example. Even if you could, this is a bad way to program.
When you need to perform an operation more than once, you need a loop. When dealing with a dynamic number of times, you have to use a loop. A condition needs to be met before you can stop performing that operation.
When you need to loop a fixed number of times, a for loop is usually best for this. An example of a for loop written in Java would be.
for (int i = 1; i <= 3; i++) {
doSomething();
}
If the language you are using supports looping using ranges, this is an even better solution than for loops. Ranges are more clear as to what you are doing and help avoid off-by-one errors. An off-by-one error is when you either skip an iteration or have an additional iteration.
Collections
When dealing with a collection of data, it is common that you need to perform an operation on each item in the collection. If you were displaying comments on a website, for each item in the collection, you would need to display it on the screen. You would accomplish this by looping over each item in the collection. When you have a collection, a for-each loop is best suited for this, or a forEach() method that takes a lambda.
// For-each loop
for (final var car : cars) {
// code to execute
}
// For each with lambda
cars.forEach(
car -> // code to execute
);
Looping over a collection could be considered the same as above, although they are different mindsets. The above loops a specific number of times, but the number of times could be considered a collection.
Doing Something While a Condition is True
Another use case for loops is needing to do something while a condition is true. If you were writing a program that needed to wait for some type of input, how would you do that? When the main() function executes, it will execute each line of code and then terminate at the end of the main() function. In order to do this, you need a loop.
while (waitingForInput()) {
// do something...
}
Another example would be having a thread running in the background that is performing a task that needs to always be running.
You would use this anytime you needed to do something while a condition was true. While loops are the best to use when you don't know the number of iterations a loop needs to execute.
Conclusion
Understanding loops is crucial for creating efficient code. Loops are a fundamental aspect of programming in many languages. However, some languages do not support loops and instead use recursion.