Working With Strings in Java
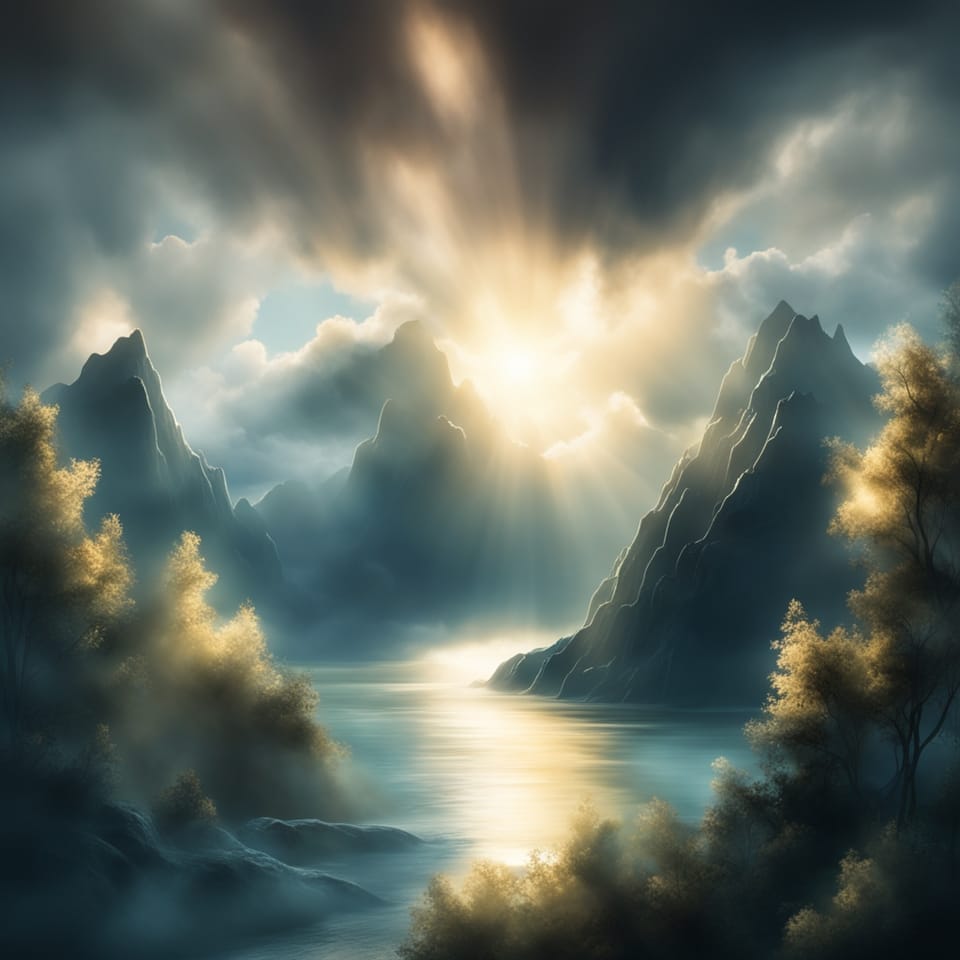
String Values
Java strings are immutable. Strings are assigned a value using double quotes.
final var s = "value";
Whenever you perform an operation on a string, the original isn't modified. Instead, a new one is created and returned. For example.
final var s = "a";
// Returns a new string in all uppercase
s.toUpperCase();
If the value of your string contains a double quote, you must escape the value.
// "Hello"
final var s = "\"Hello\"";
Null Strings
Strings are classes, so they can be assigned the value of null. A null String isn't the same value as an empty String. The two variables in the following example are two different values.
final String empty = "";
final String nullString = null;
String Pools
There are two ways to assign a value to a string. The first is using double quotes.
final var s = "a";
This is generally how you should assign a value to a string. The second way is to use the new keyword. This actually creates a new String.
final var s = new String("a");
When working with strings, there is actually a pool of strings. Java will cache a string's value and reuse it. By using new String(""), Java will create a new string and not use one from cache. If you were to put the first example in a loop and call it 1,000 times, only one string would be created. If you were to do the same thing with the second example, each iteration would create a new string.
Combining Strings
You can combine strings using +. You can combine either literal string values or string variables.
public String sayHello(
final String firstName,
final String lastName) {
return "Hello " + firstName + " " + lastName;
}
// Hello Jim Smith
final var greeting = sayHello("Jim", "Smith");
Building Strings
Sometimes you need to build a string over multiple lines of code. You can use the += operator to do this.
var s = "a";
s += "b";
s += "c";
Although this works, you shouldn't use += when building strings. Building strings with += can have a major performance impact. Each time += is used, a new string is created.
When building strings, use either the StringBuffer or StringBuilder class. Both classes extend AbstractStringBuilder so they share the same API (Application Programming Interface). StringBuffer is synchoronized, so it is thread-safe. Use this only if you are dealing with multi-thread code. If you aren't dealing with multi-threaded code, use StringBuilder. StringBuilder isn't synchronized, so you will get better performance than StringBuffer.
You can build strings using these two classes with the append() method.
final var s = new StringBuilder();
s.append("a");
s.append("b");
s.append("c");
String Equality
Just like numbers, you can use the == operator for equality and the != operator for inequality. Since strings are classes, these operators check the instance of the string, not the value of the string. You can have a string with the same value, but checking for equality with == could return false. For example.
public boolean isEqual(
final String a,
final String b) {
return a == b;
}
This could result in either true or false, even if the values of a and b are the same. To check for equality or inequality on a string's value, you use the equals() method.
final var isEqual = a.equals(b);
final var notEqual = !a.equals(b);
This checks to see if the value is equal instead of if it is the same instance. This can be a gotcha for new Java developers.
CharSequence Interface
The String class and other String like classes such as StringBuffer and StringBuilder implement the CharSequence interface. Classes that implement this interface are not guaranteed to be immutable. You generally will not use this interface. It is just something to be aware of as a Java developer. If you need another String like class, such as StringBuffer, it would be better to use that type instead.
Conclusion
There is a lot you can do with Java strings. This article covers the basics of working with strings.